Executing Pythons First Program in 14 IDEs
Running Your First Python Program: “Hello, World!”
❉ Introduction
Welcome to your journey with Python! By now, you have already learned about Python and its applications, and you’ve chosen an IDE (Integrated Development Environment) for your coding experience. The next step is to write and run your first Python program.
In this post, we’ll walk you through the process of creating and running the classic “Hello, World!” program using Python. This is the first program every programmer writes in a new language, and it’s a great starting point to understand how to write code, execute it, and view the output.
We’ll cover how to write and run “Hello, World!” in 14 different IDEs and text editors, providing step-by-step instructions for each. We’ll also discuss which IDE might be best for different purposes, so you can make an informed decision on which tool to use for your Python projects.
❉ What is “Hello, World!”?
The “Hello, World!” program is a simple program that outputs the text “Hello, World!” to the console. It’s typically the first program a new programmer writes in any language. Although this program doesn’t do anything complex, it serves as an introduction to the syntax and structure of the language and the process of running a program.
In Python, writing “Hello, World!” is as simple as this:
print("Hello, World!")
The print()
function is built into Python and sends whatever is inside the parentheses to the screen (or “console”), making it perfect for a beginner’s first program.
❉ Setting Up Python
Before you can write your first Python program, ensure that Python is installed on your computer. If you haven’t done so yet, you can follow our previous post about installing Python.
Once Python is installed, you can use different IDEs (Integrated Development Environments) to write, edit, and run Python code. Let’s take a closer look at 14 popular IDEs you can use to run “Hello, World!” and how to set them up.
❉ Running Your First Python Program in 14 Different IDEs
Python offers various Integrated Development Environments (IDEs) and text editors, each with its own set of features. Whether you’re a beginner or an experienced developer, choosing the right environment can enhance your coding experience. In this guide, we’ll walk through how to write and execute the classic “Hello, World!” program in 14 popular IDEs.
These IDEs range from simple environments like IDLE to more advanced setups like PyCharm and VS Code, each providing unique features tailored to different needs. Let’s explore each of these and get started with your first Python program.
➤ Running “Hello, World!” in IDLE
IDLE is the default IDE that comes pre-installed with Python. It’s very simple and great for beginners.
Open IDLE (search for it in your Start Menu or Applications folder).
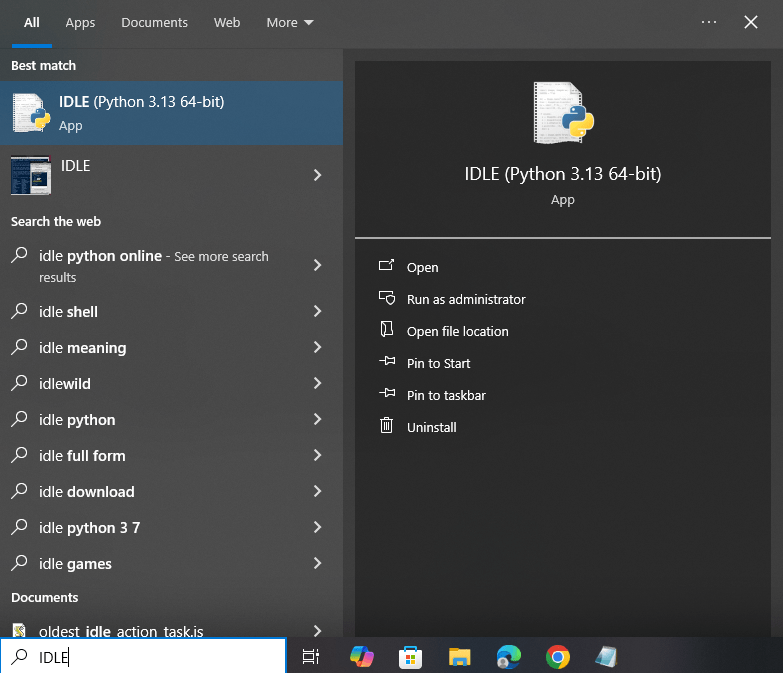
You’ll see the Python Shell, which is an interactive console.
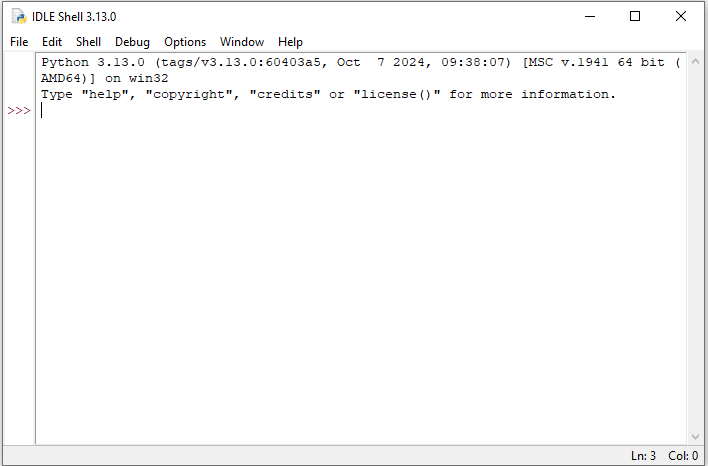
You can directly type print("Hello, World!")
into the shell.
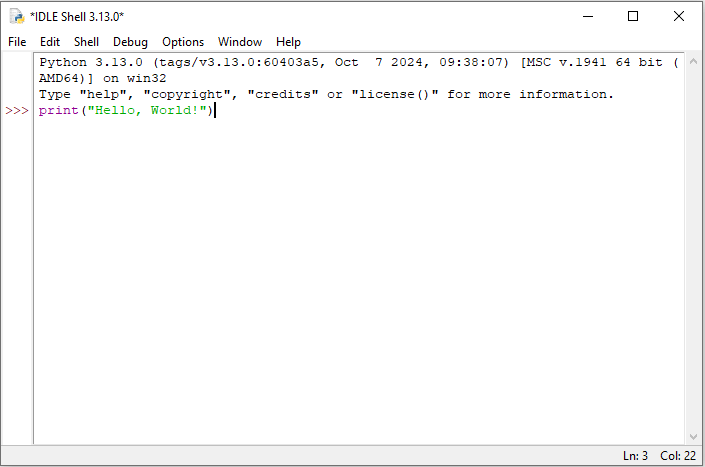
Press Enter.
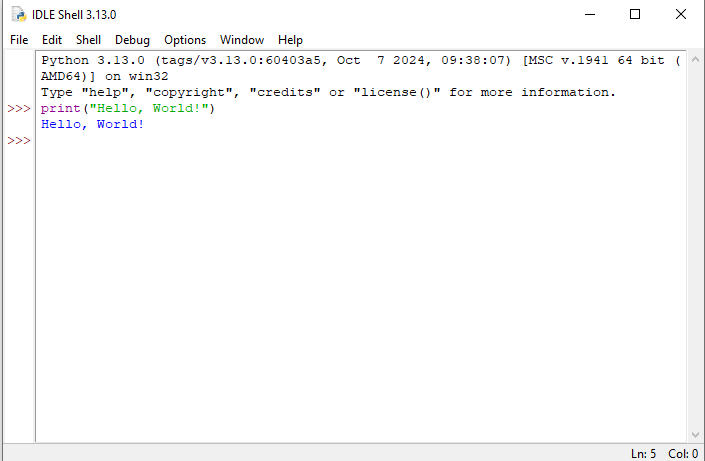
⇒ Alternatively, you can open a new file by selecting File > New File.
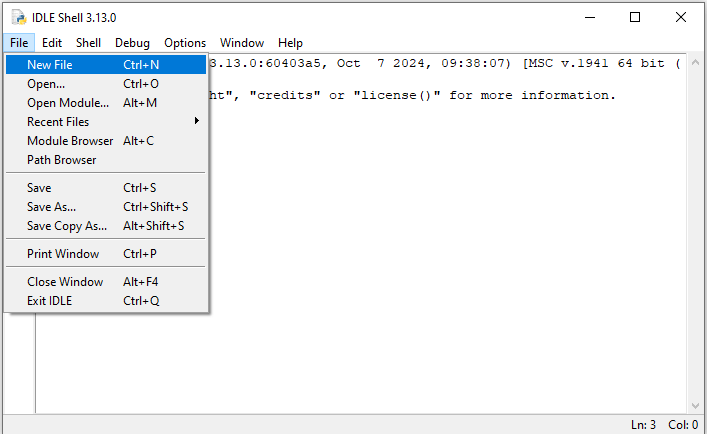
Type your code.
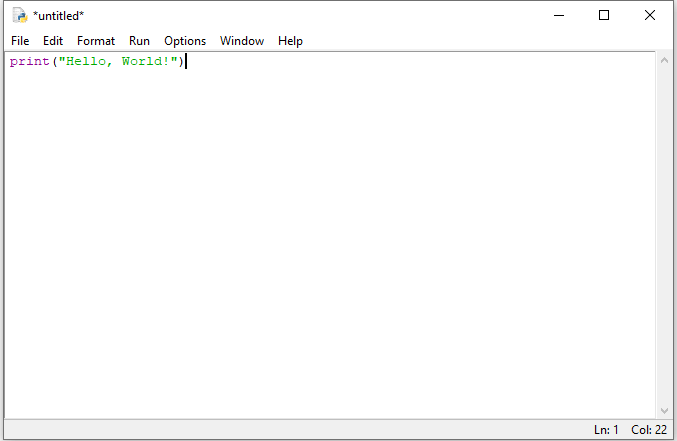
Save it with a .py
extension.
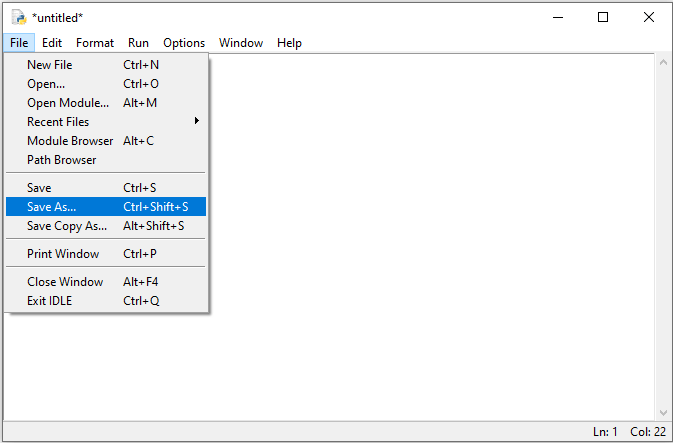
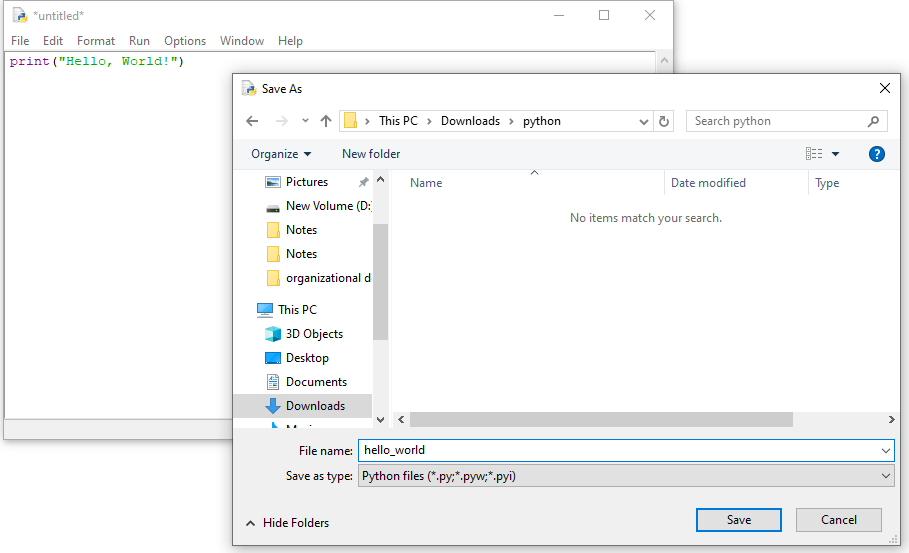
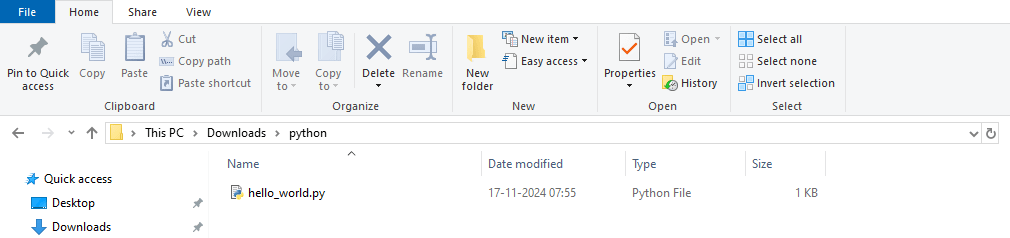
After that, click Run > Run Module (or press F5) to execute the code.
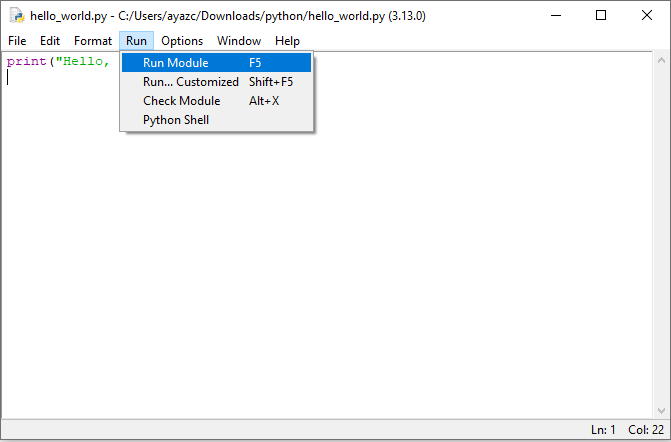
Output:
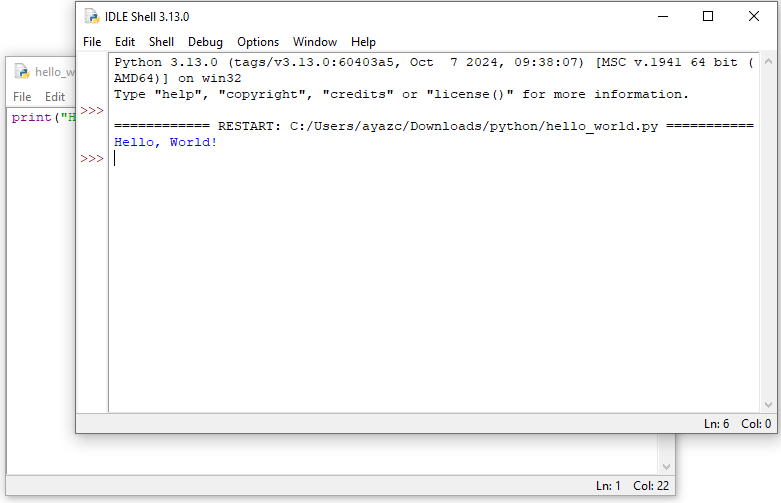
Why Use IDLE? IDLE is very beginner-friendly, with no need to configure anything. It’s useful for simple scripts and learning the basics of Python.
➤ Running “Hello, World!” in Command Prompt/Terminal
Command Prompt/Terminal is a simple text-based interface that allows you to interact with your operating system, and it can be used to run Python scripts directly from the command line.
Open the Command Prompt (Windows) or Terminal (macOS/Linux).
Start the Python interpreter by typing python
(or python3
depending on your system).
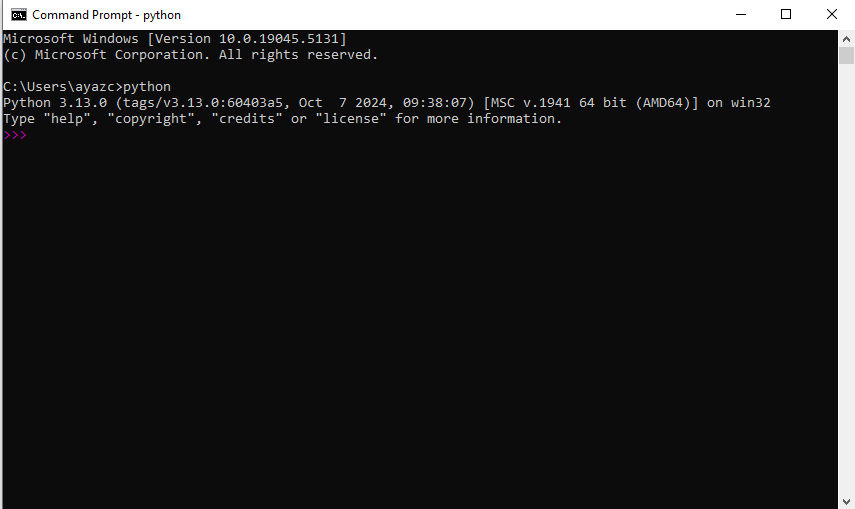
Directly type the code: print("Hello, World!")
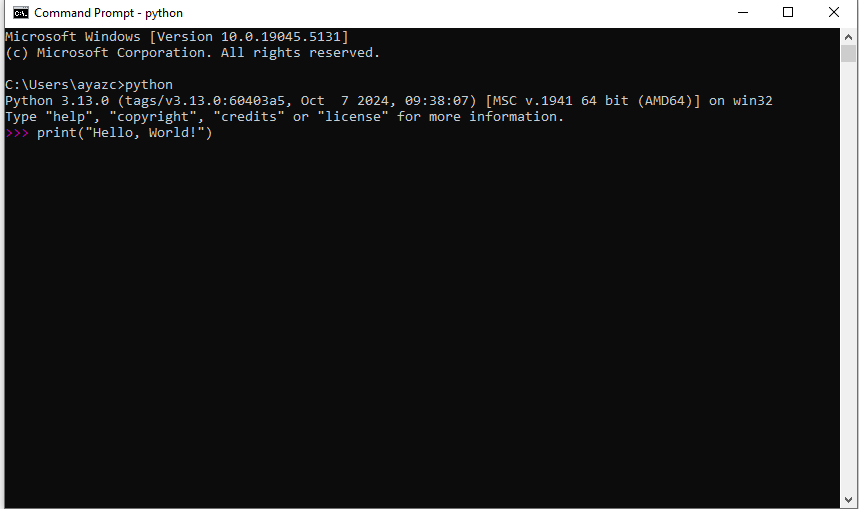
Output
Press Enter to see the output.
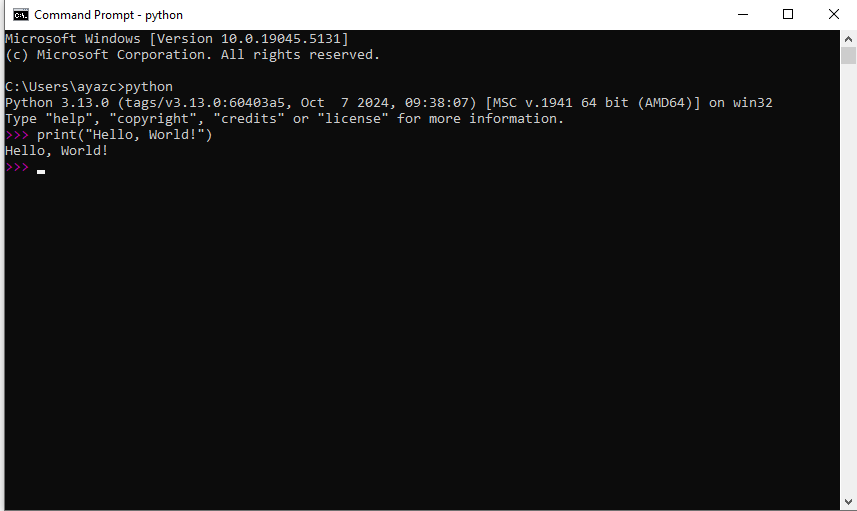
Why Use Command Prompt/Terminal?
The Command Prompt (Windows) or Terminal (macOS/Linux) provides a quick and efficient way to run Python code without needing to open an external IDE. It’s great for quick scripts or when you’re already working in the terminal for other tasks.
➤ Running “Hello, World!” in Notepad++
Notepad++ is a lightweight text editor that you can use to write Python scripts.
Download and Install Notepad++:
- Visit the Notepad++ official website and download the installer for your operating system.
- Follow the installation prompts to install Notepad++ on your computer.
Open Notepad++ and type the code: print("Hello, World!")
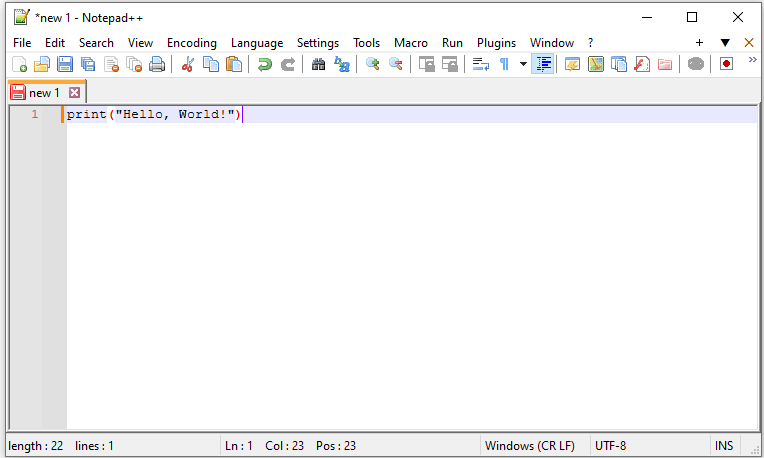
Save the file with a .py
extension.
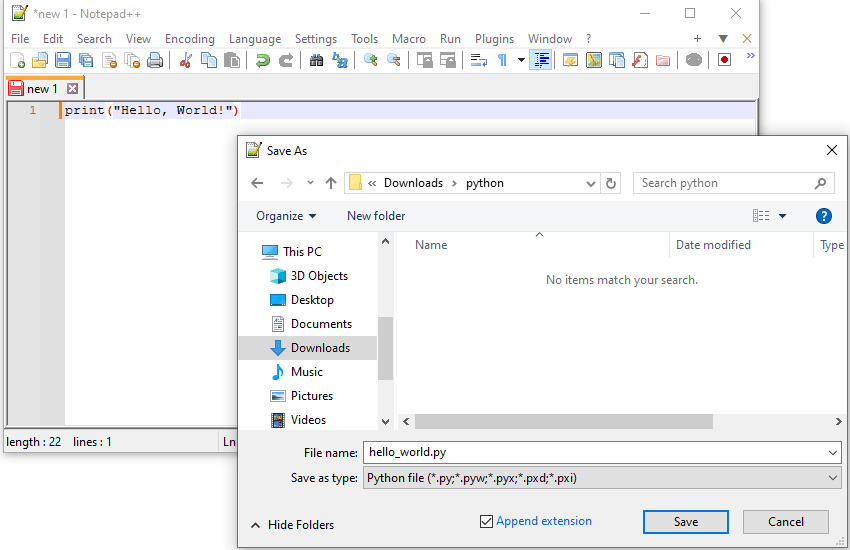
Open a Command Prompt/Terminal, navigate Navigate to the directory where you saved the hello_world.py
file using the cd
command.
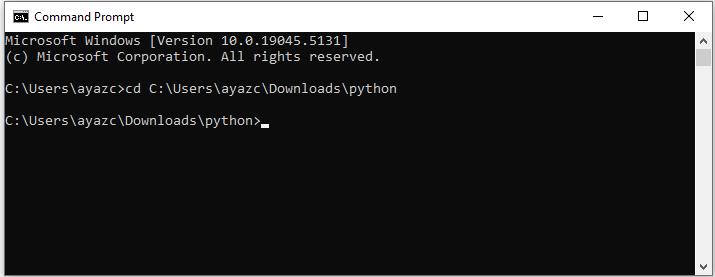
Execute the file by typing python filename.py
.
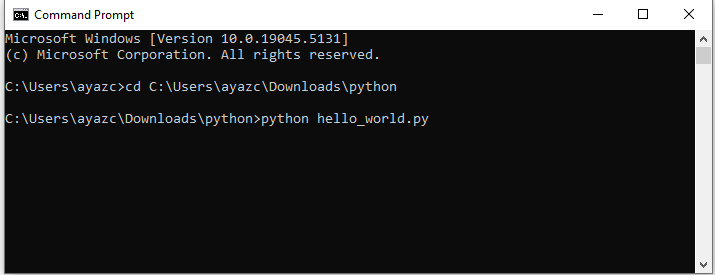
Output
Press Enter to see the output.
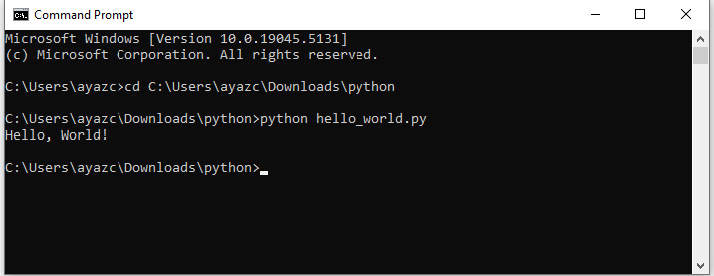
Why Use Notepad++?
Notepad++ is a simple, fast text editor that supports Python syntax highlighting. It’s perfect for quick edits and small Python scripts, making it ideal for beginners or anyone working on simple projects.
➤ Running “Hello, World!” in Thonny
Thonny is an easy-to-use IDE for beginners and is perfect for learning Python. It provides a simple interface and integrated debugger, which can help new programmers step through their code.
Download Thonny:
- Go to the official Thonny website.
- Click on the appropriate download link for your operating system (Windows, macOS, or Linux).
Install Thonny:
- For Windows: Run the downloaded
.exe
file and follow the on-screen instructions to complete the installation. - For macOS: Open the downloaded
.dmg
file, drag Thonny to the Applications folder, and launch it. - For Linux: Use your package manager to install Thonny or follow the instructions on the website.
Verify Installation:
- Open Thonny from your Start Menu, Applications folder, or Terminal. You should see the Thonny IDE interface.
Launch Thonny.
- On Windows/macOS: Double-click the Thonny icon.
- On Linux: Type
thonny
in the terminal and press Enter.
In the Editor window (the top section of Thonny), type the following code print("Hello, World!")
.
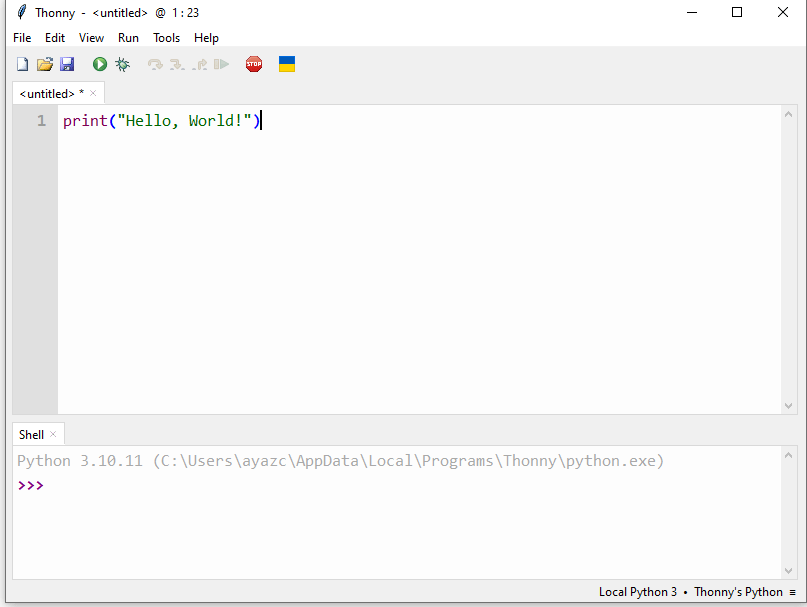
Save the file:
- Click File > Save As.
- Choose a location, and save the file with a
.py
extension (e.g.,hello_world.py
).
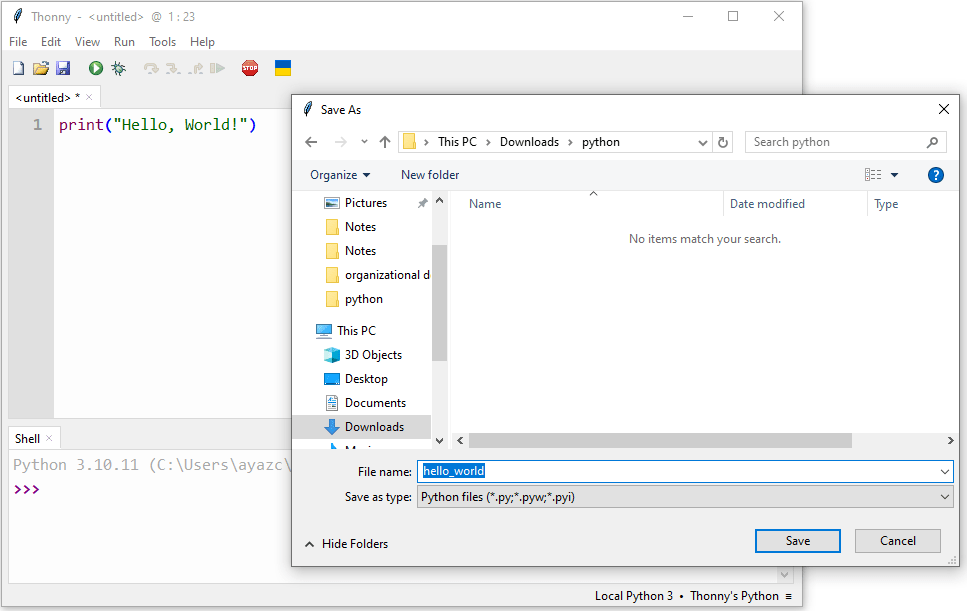
Run your script:
- Click the Run button (green triangle) in the toolbar or press F5 on your keyboard.
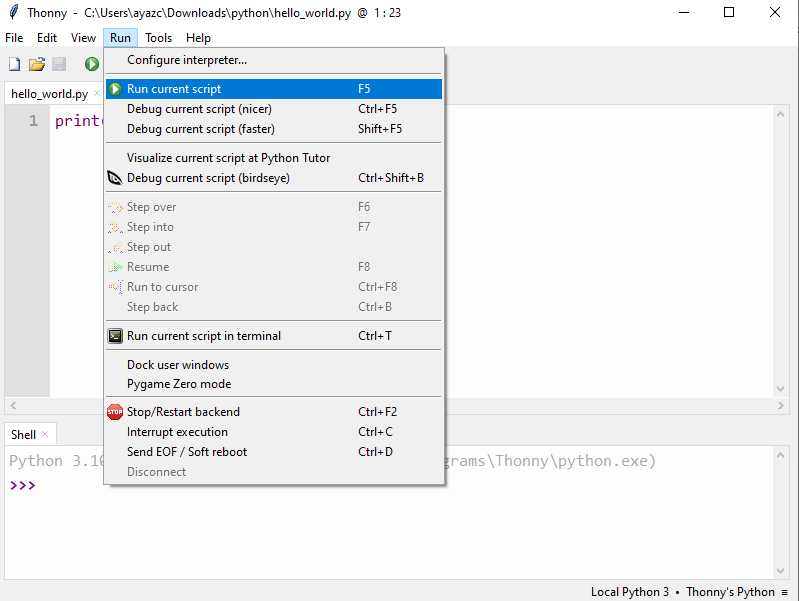
Output
The output will appear in the Shell (the bottom section of Thonny) and should look like this.
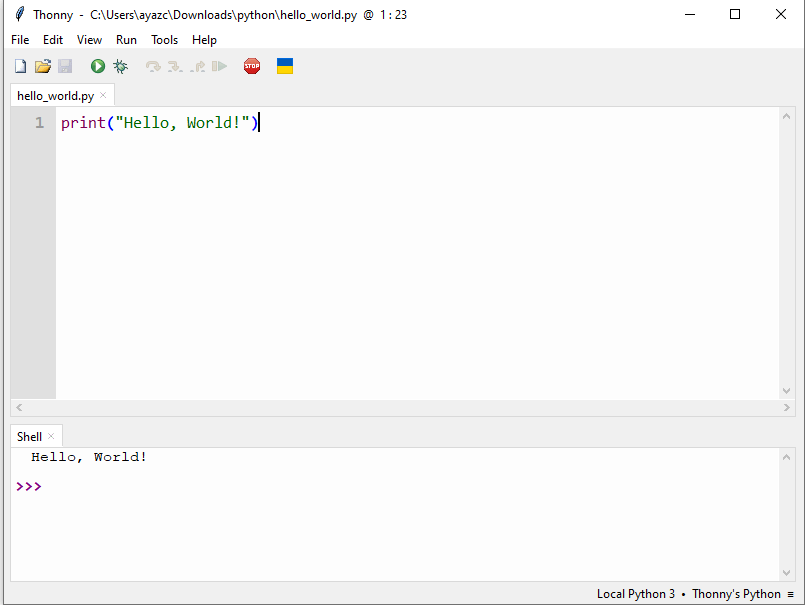
Why Use Thonny? Thonny is simple yet powerful, with features like debugging and variable tracking that are perfect for beginners who want to understand how their code works step by step.
➤ Running “Hello, World!” in Jupyter Notebook
Jupyter Notebook is a popular tool for data science and machine learning. It allows you to write code in cells and run them one at a time. It’s ideal for working with large data sets and visualizing outputs.
Install Jupyter Notebook:
If you haven’t already installed Jupyter Notebook, you can do so by opening a terminal (or command prompt) and running: pip install notebook
.
This will install Jupyter Notebook on your machine.
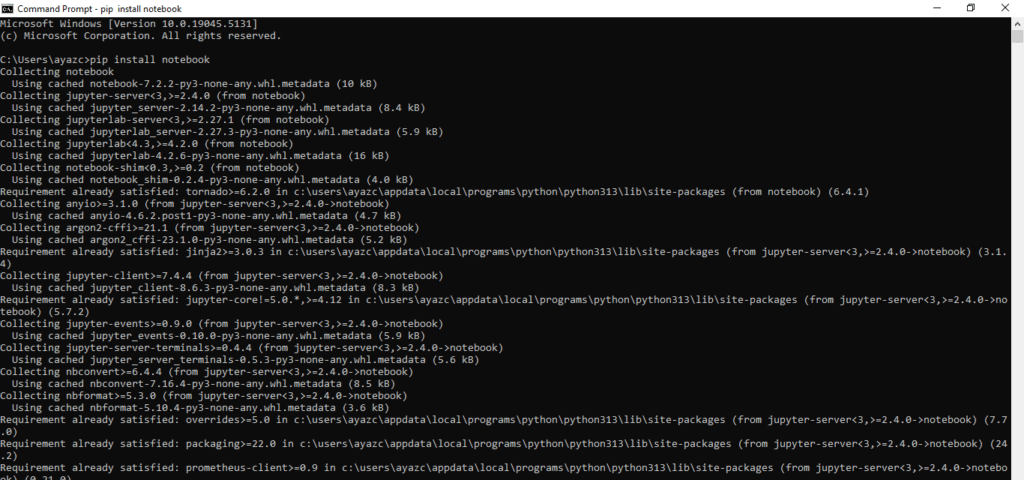
Launch Jupyter Notebook:
- Run the following command to start Jupyter Notebook:
jupyter notebook
.
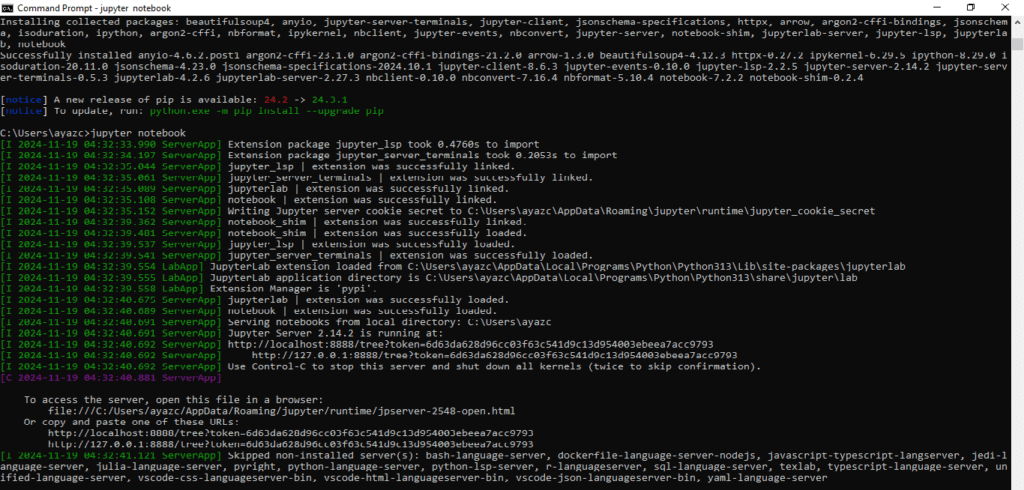
- This command will open Jupyter Notebook in your default web browser. You should see the Jupyter Notebook dashboard with various options to create notebooks.
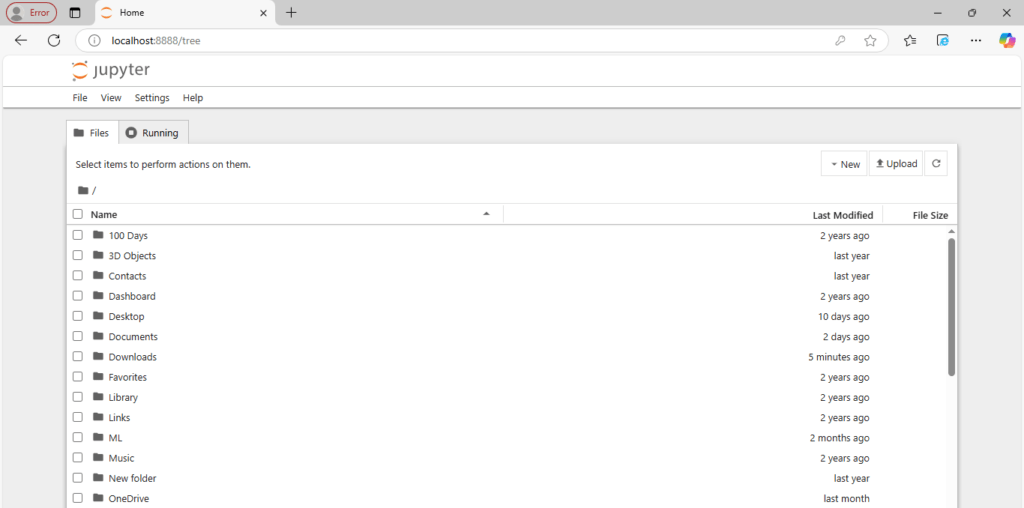
From the Jupyter Notebook dashboard:
- Click on New and select Python 3. This will create a new Python notebook file (
.ipynb
).
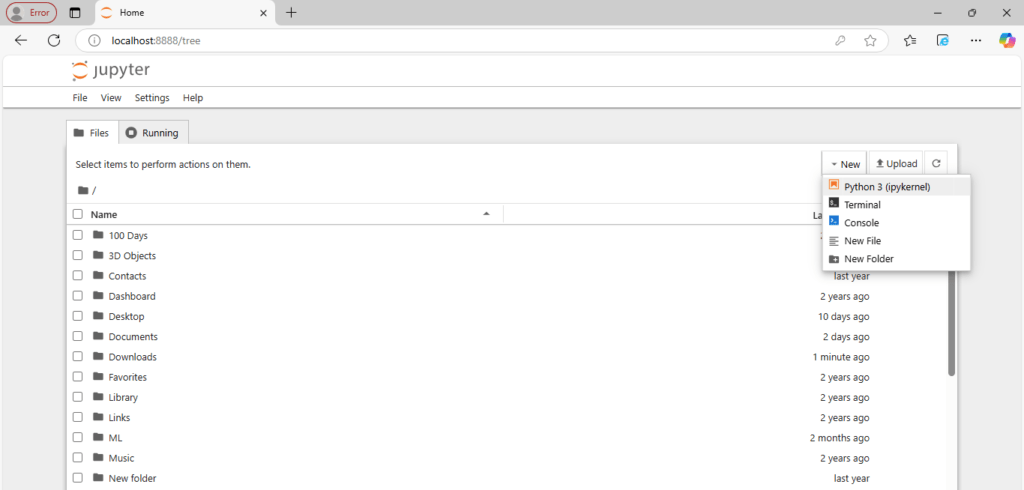
In the new notebook:
- Click on a cell and type the following code to print “Hello, World!”:
print("Hello, World!")
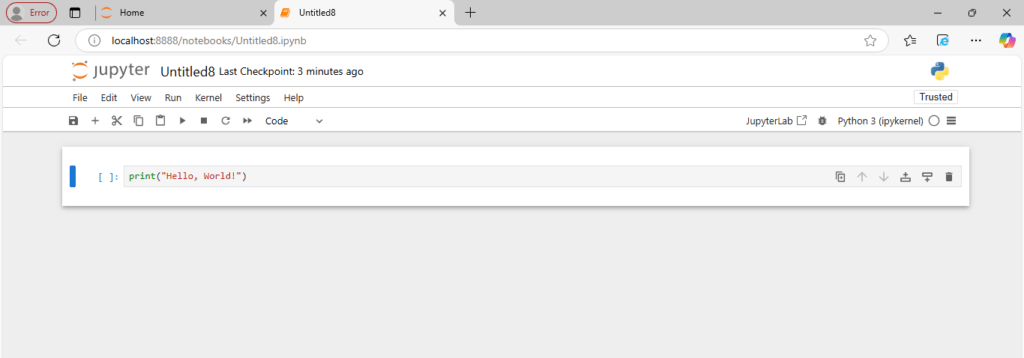
- You can run the code in a cell by clicking on the cell and then pressing Shift + Enter. This will execute the cell and display the output below it.
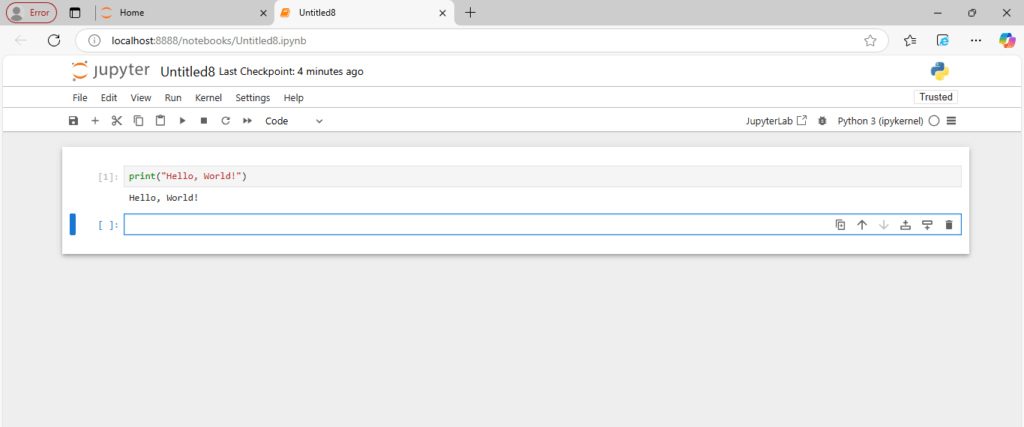
To save your notebook:
- Go to File > Save Notebook As…
- This will save your notebook with a
.ipynb
file extension. You can give it a name likehello_world.ipynb
.
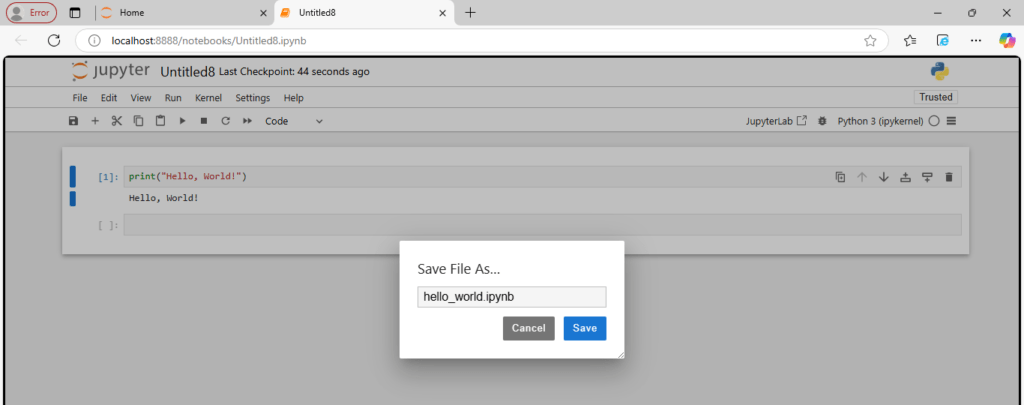
Why Use Jupyter Notebook? Jupyter is great for data analysis, visualization, and learning. It’s interactive, so you can experiment with code and immediately see the output.
➤ Running “Hello, World!” in PyCharm
PyCharm is a professional-grade IDE used by Python developers for large projects. It’s feature-rich with powerful tools for coding, debugging, and project management.
Download and Install PyCharm
- Open a web browser and visit the official PyCharm website.
- Click the Download button.
- Choose between the Professional version (paid, with advanced features) or the Community version (free and suitable for beginners).
- Run the installer file you downloaded.
- Follow the installation wizard:
- Select the installation path.
- Check the box for adding PyCharm to your system PATH and create a desktop shortcut (optional).
- Click Install and wait for the process to complete.
- After installation, click Finish and launch PyCharm.
Configure PyCharm
- When you launch PyCharm, you’ll be prompted to configure settings (like themes and plugins). Use the defaults for now.
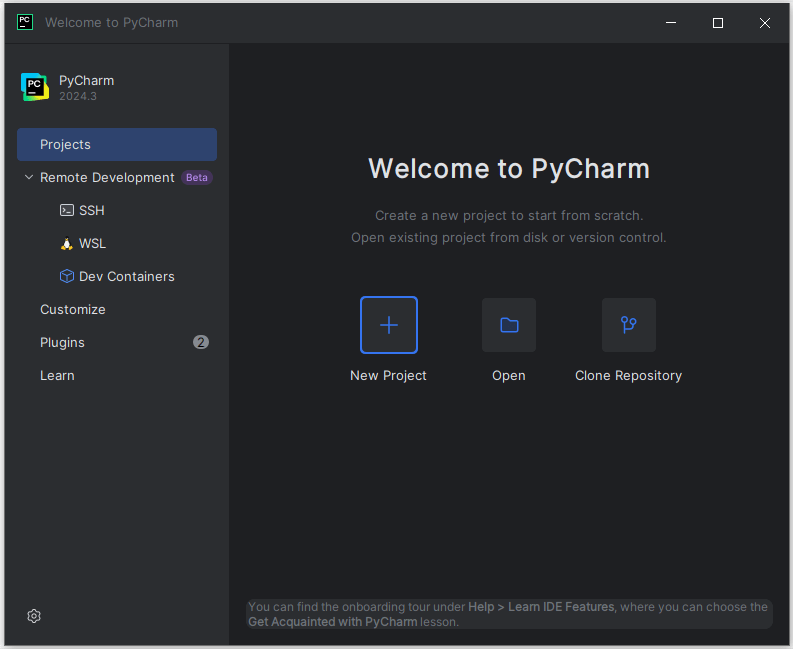
- Click on New Project. In the window that appears: Specify the project location .
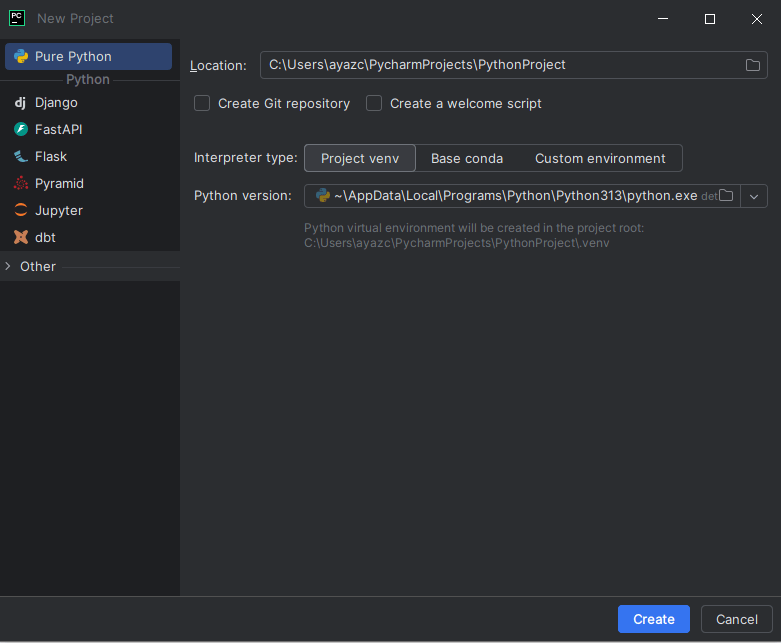
- Set Up the Python Interpreter:
- Go to File > Settings > Project: <project name> > Python Interpreter.
- Choose your Python installation from the list or specify its location manually.
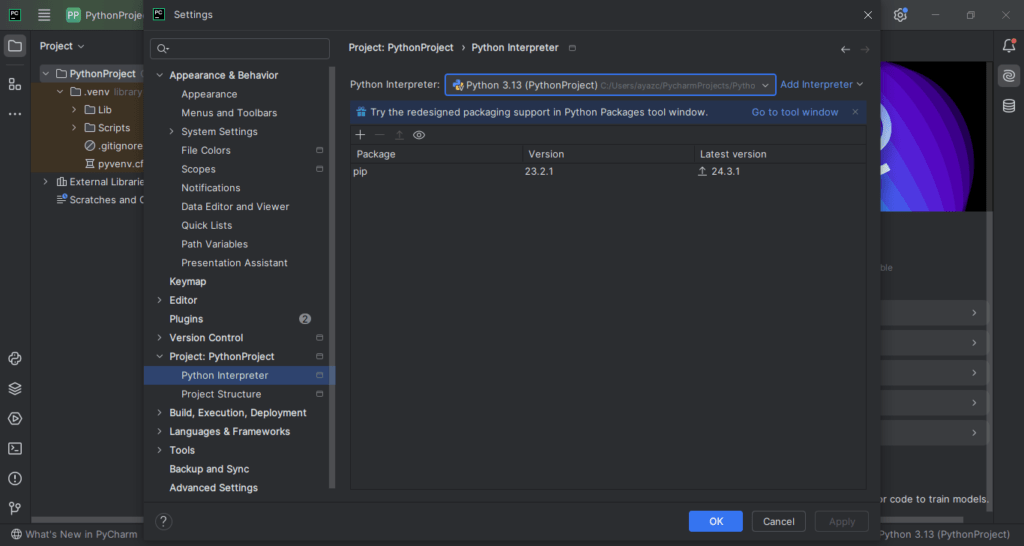
Write the “Hello, World!” Program
- Right-click on the project name in the Project tool window.
- Select New > Python File.
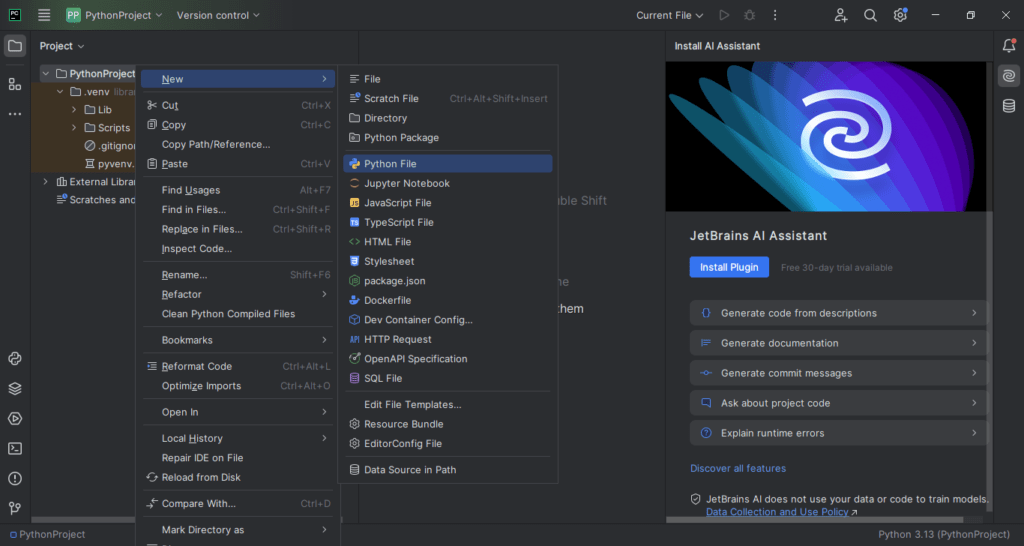
- Enter the file name, e.g.,
hello_world
. - In the newly created Python file, type the following code:
print("Hello, World!")
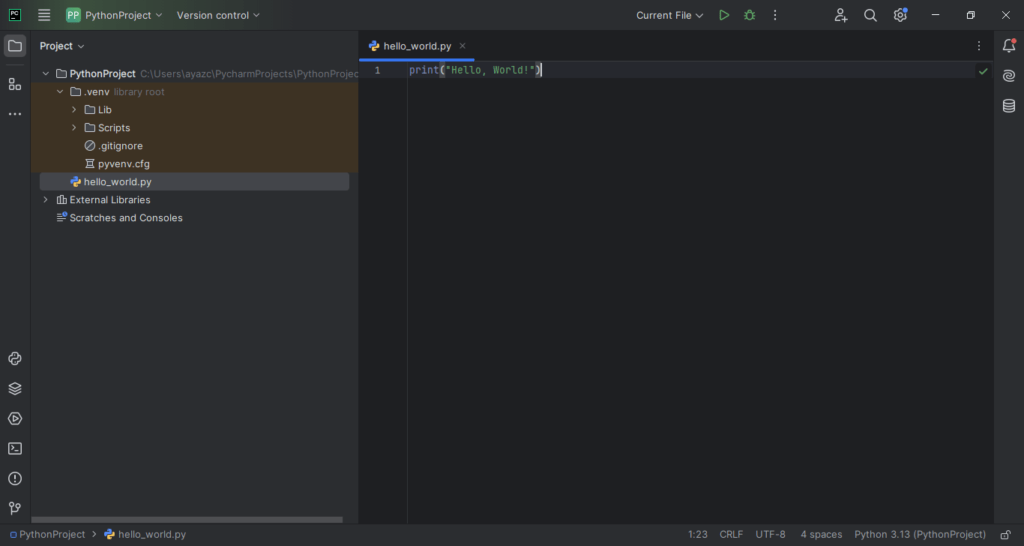
Run the Program
- Right-click inside the code editor window and select Run ‘hello_world’.
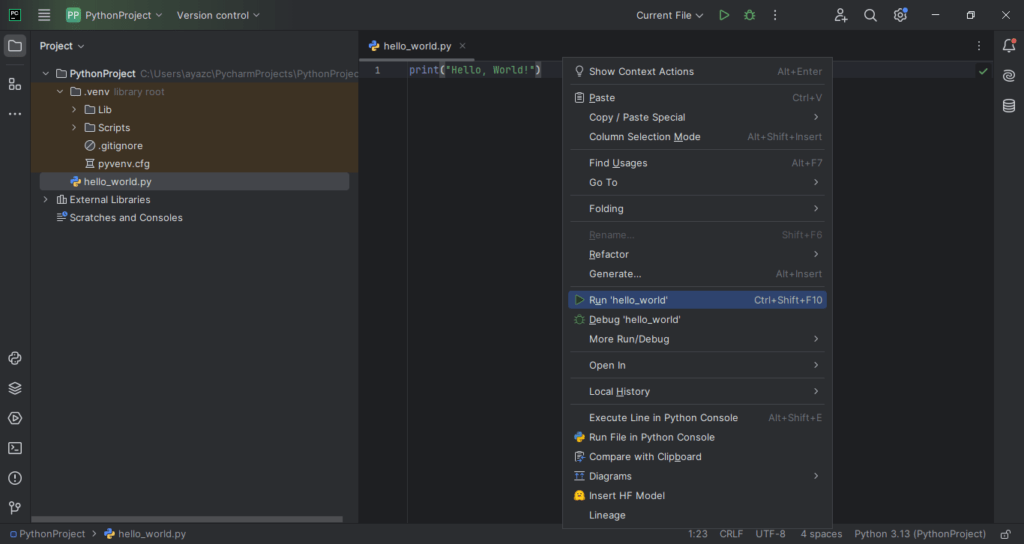
- Alternatively, you can go to the top-right corner and click the Run button.
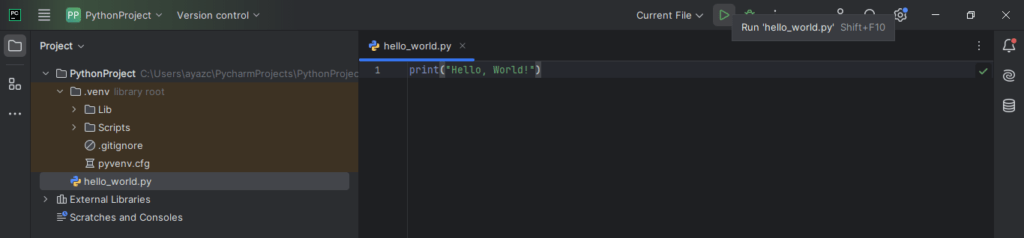
Output
The Run tool window will appear at the bottom of the screen.You’ll see the output
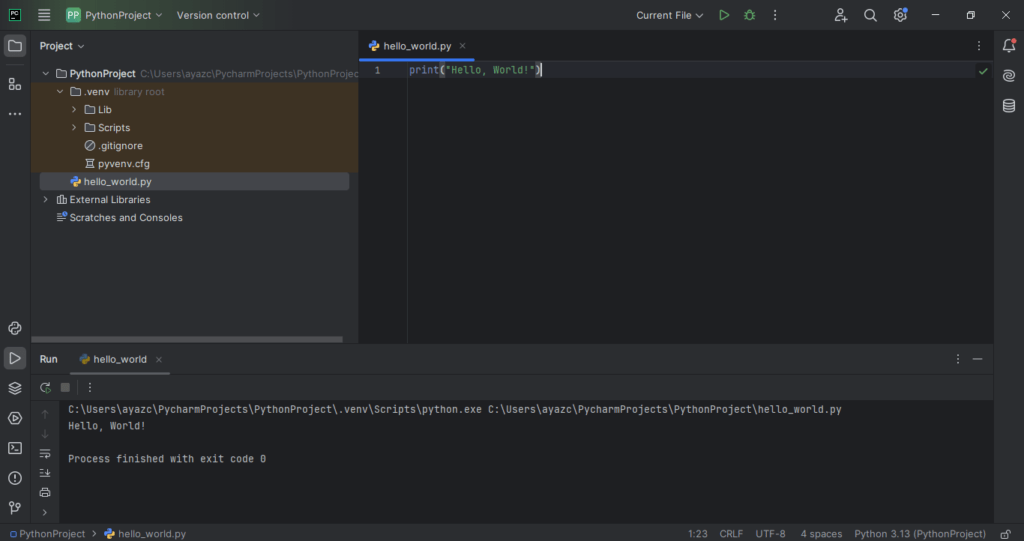
Why Use PyCharm? PyCharm is perfect for developers working on large Python projects or with complex libraries. It provides advanced debugging, testing, and version control tools.
➤ Running “Hello, World!” in Visual Studio Code (VS Code)
Visual Studio Code is a lightweight, customizable code editor. It’s a great choice for Python development, with an extensive marketplace for extensions.
Download VS Code:
- Go to the official VS Code website.
- Click the Download button for your operating system (Windows, macOS, or Linux).
Install VS Code:
- Run the installer file you downloaded.
- Follow the installation wizard:
- Accept the license agreement.
- Choose the installation location.
- Check options like “Add to PATH” (recommended) for easier command-line use.
- Click Install, and then click Finish when done.
- Launch Visual Studio Code from the start menu or applications folder.
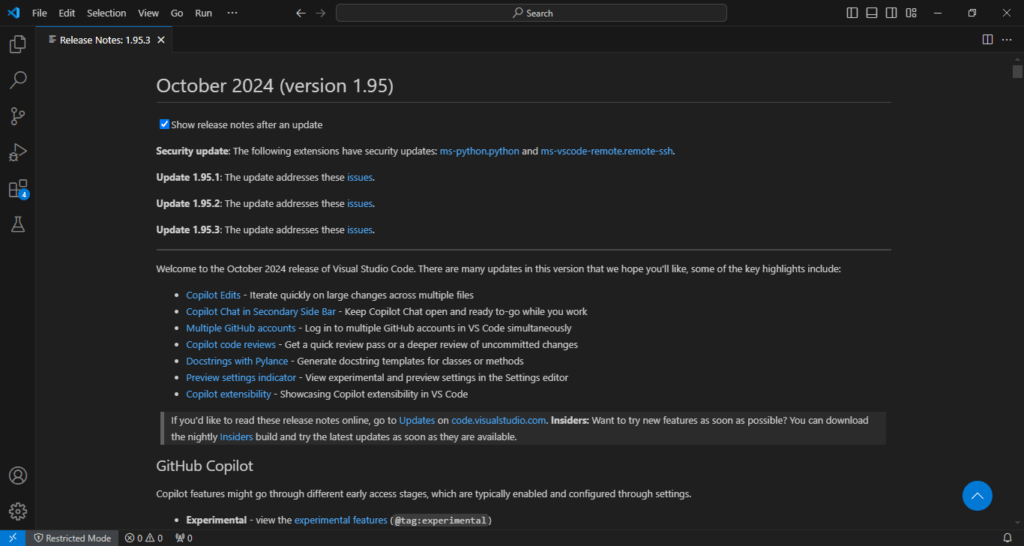
Install the Python Extension for VS Code
- Click on the Extensions icon in the Activity Bar on the left (it looks like four squares).
- In the search bar, type Python.
- Click the Install button next to the official Python extension by Microsoft.
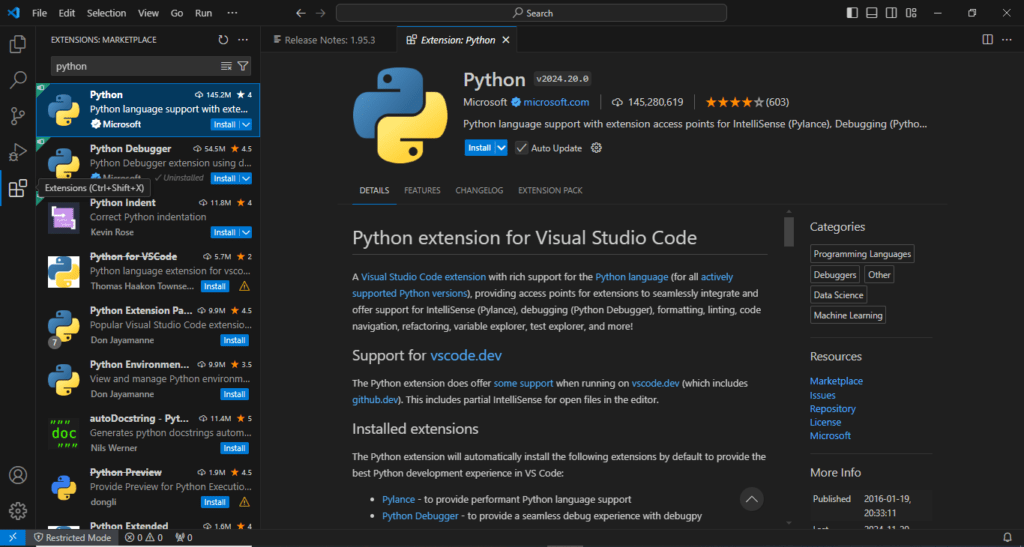
Create a New Python File
- In VS Code, click File > New File (or press
Ctrl + N
).
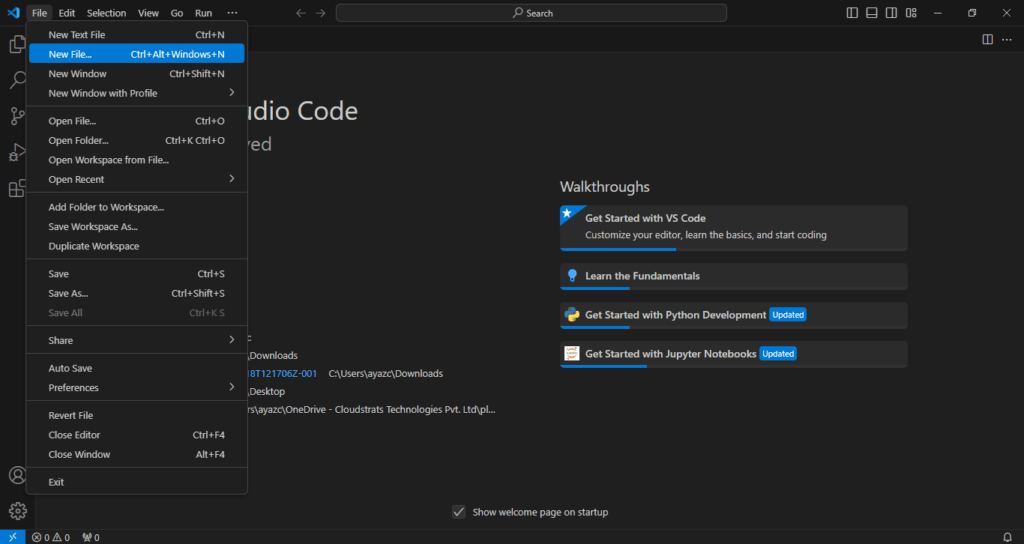
- Click File > Save As.
- Name the file
hello_world.py
and save it in a folder of your choice.
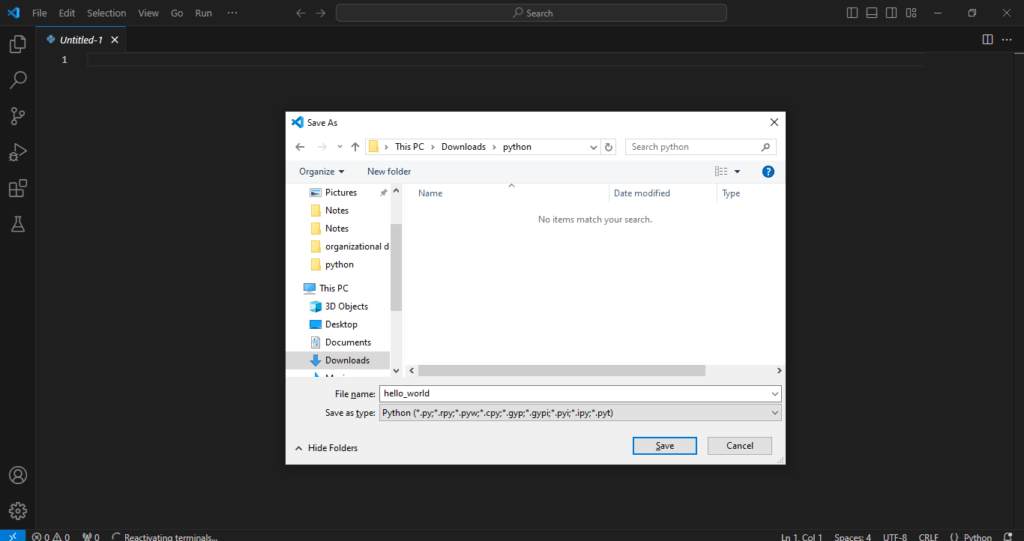
- Ensure the file has the
.py
extension, indicating it’s a Python file.
Write the “Hello, World!” Program
In the editor, type the following code: print("Hello, World!")
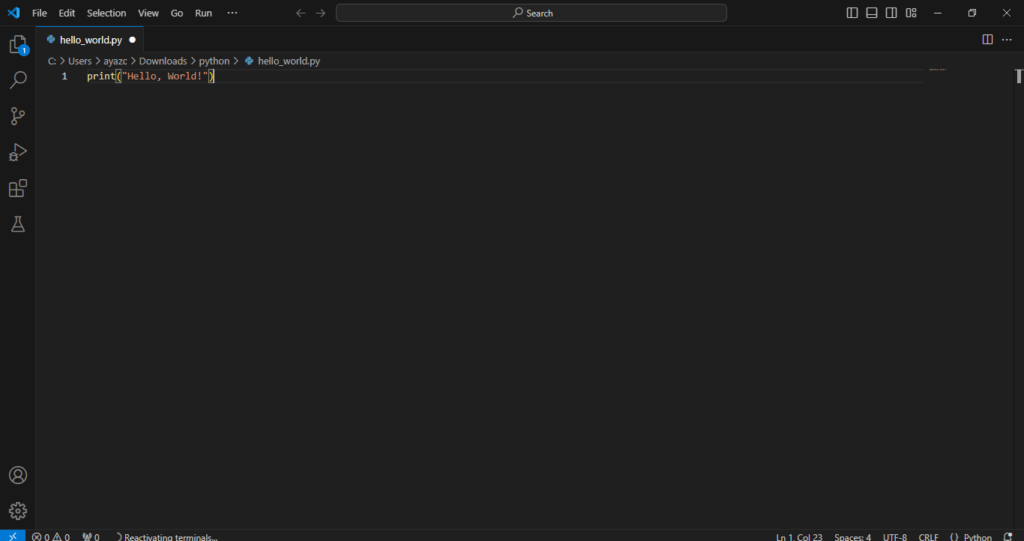
Run the Program
- Click Terminal > New Terminal from the menu, or press
Ctrl + `
(backtick).
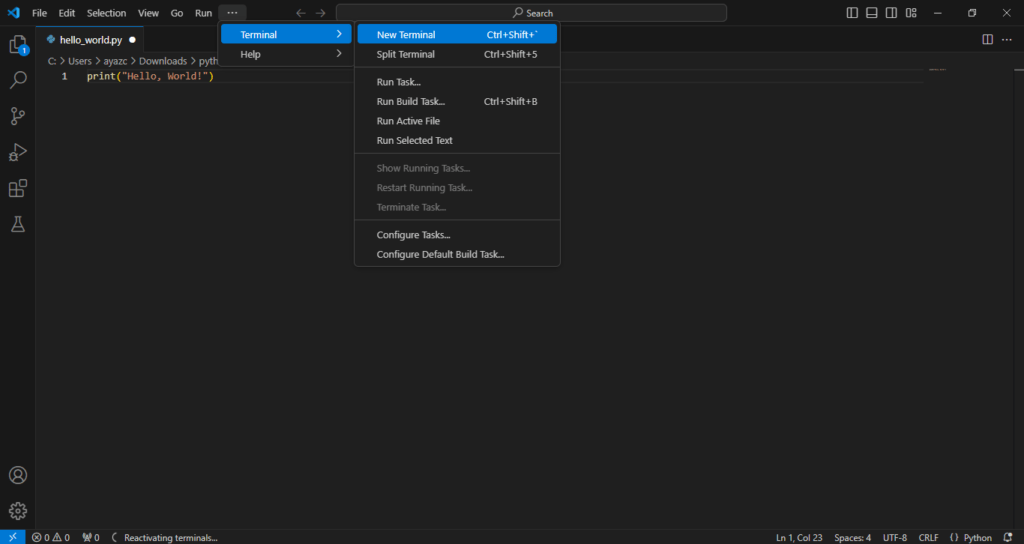
- Run the program by typing:
python hello_world.py
- Press Enter.
Output
In the terminal, you should see the output:
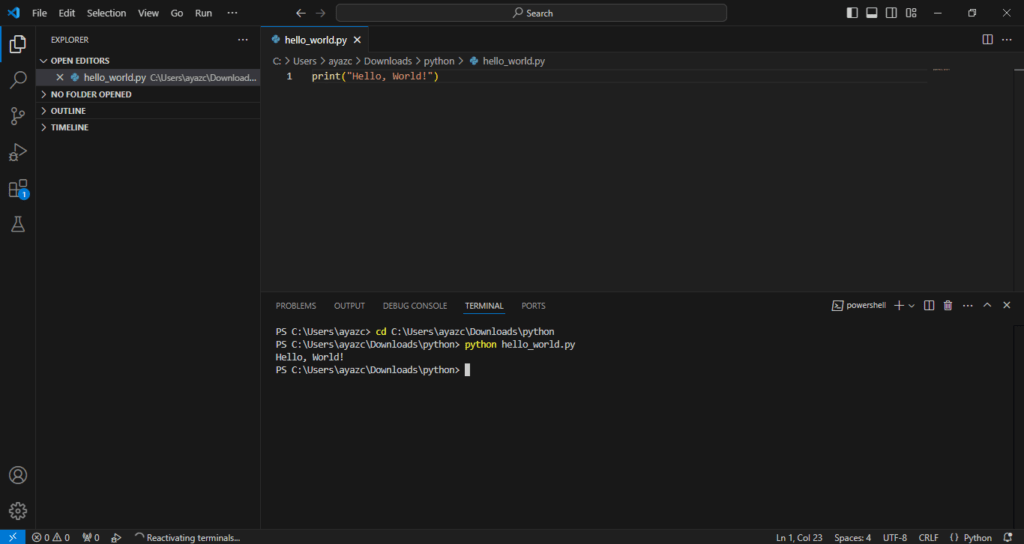
Why Use VS Code? VS Code is fast, customizable, and has powerful extensions. It’s perfect for Python developers who want an editor that’s not too heavy but still packed with features.
➤ Running “Hello, World!” in Anaconda Navigator
Anaconda Navigator is a GUI tool for managing Python environments, packages, and IDEs like Jupyter and Spyder.
Download Anaconda:
- Go to the official Anaconda website.
- Download the version for your operating system (Windows, macOS, or Linux).
Install Anaconda:
- Run the downloaded installer.
- Follow the on-screen instructions, ensuring the “Add Anaconda to my PATH environment variable” option is selected (if available).
- Complete the installation.
Launch Anaconda Navigator
- Open Anaconda Navigator (search for “Anaconda Navigator” in your system’s Start Menu or Applications).
- Anaconda Navigator provides a graphical interface for managing environments, packages, and IDEs like Jupyter Notebook and Spyder.
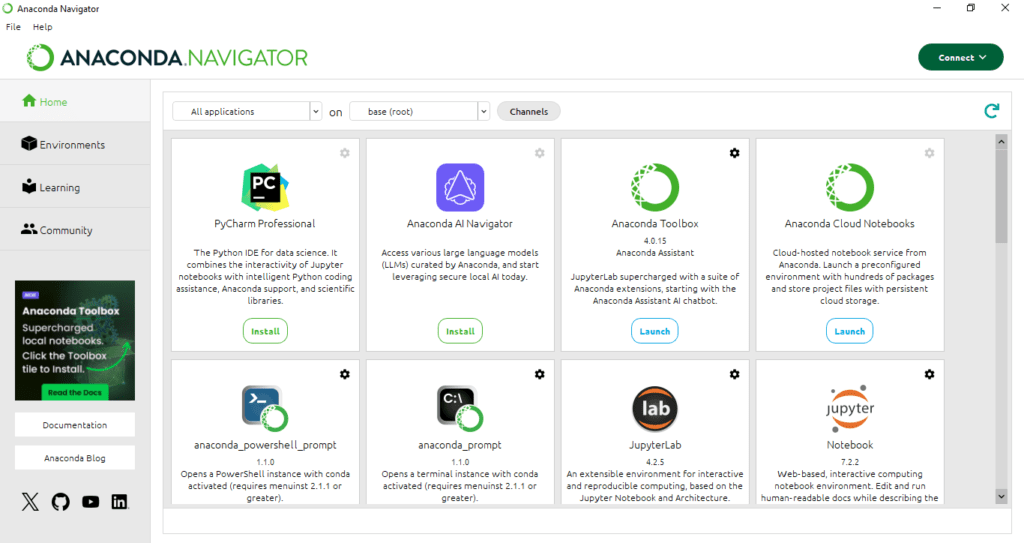
In Anaconda Navigator, locate Jupyter Notebook in the application list and click Launch.
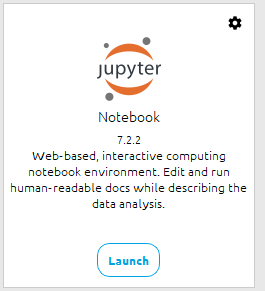
- Jupyter Notebook will open in your default web browser.
- Create a new notebook:
- Click New > Python 3 (ipykernel) in the top-right corner.
- In the first cell of the notebook, type:
print("Hello, World!")
- Press Shift + Enter to execute the cell.
Output: Hello, World!
In Anaconda Navigator, locate Spyder in the application list and click Launch.
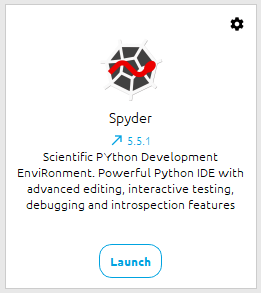
- Once Spyder opens, you will see the editor and the console.
- In the editor, type the following code:
print("Hello, World!")
- Save the file with a
.py
extension (e.g.,hello_world.py
). - Press the Run button (green triangle icon) or hit F5 to execute the script.
Output: Hello, World!
Why Use Anaconda Navigator? Anaconda Navigator is great for data scientists, as it lets you manage Python environments and packages easily. It integrates well with tools like Jupyter and Spyder.
➤ Running “Hello, World!” in Spyder
Spyder is an IDE designed for scientific computing with support for data analysis and visualization.
Install Spyder using pip:
- If you haven’t already installed
spyder
, you can do so by opening a terminal (or command prompt) and running:pip install
.spyder
This will installspyder
on your machine.
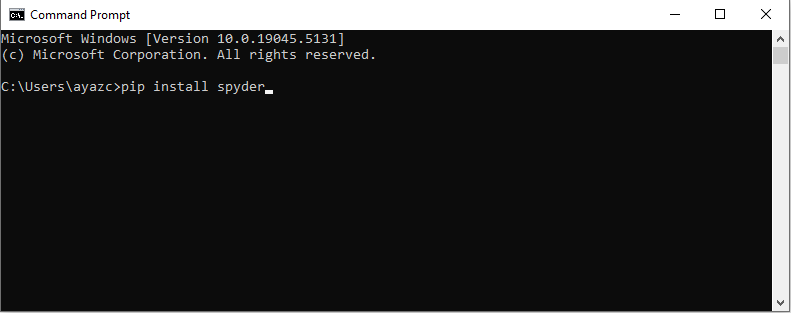
Check Version Using pip
- In the terminal or command prompt, type:
pip show spyder
This will display information about the installed Spyder package, including its version.
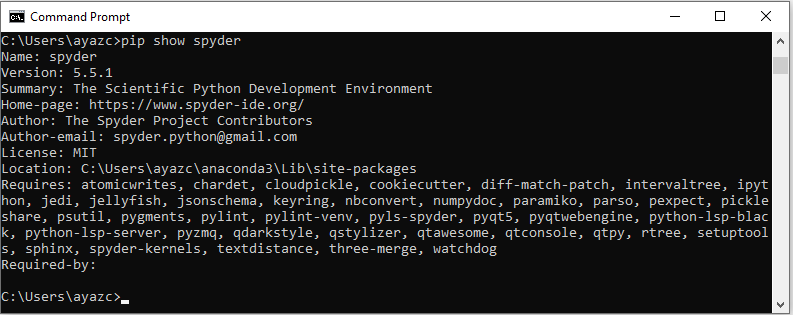
Launch Spyder
- Type
spyder
and press Enter to launch the IDE.
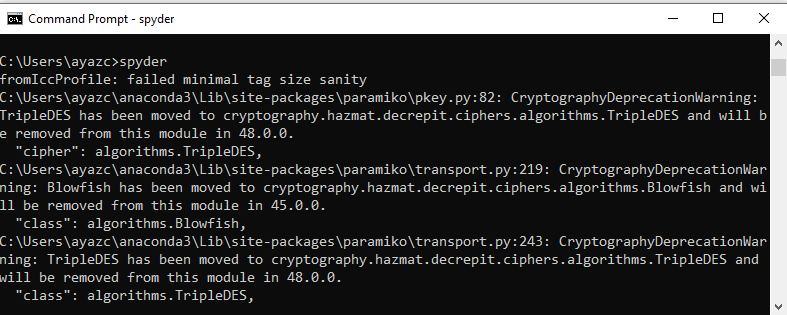
Write “Hello, World!” Program
- Once Spyder opens, locate the Editor panel on the right side (where you write code).
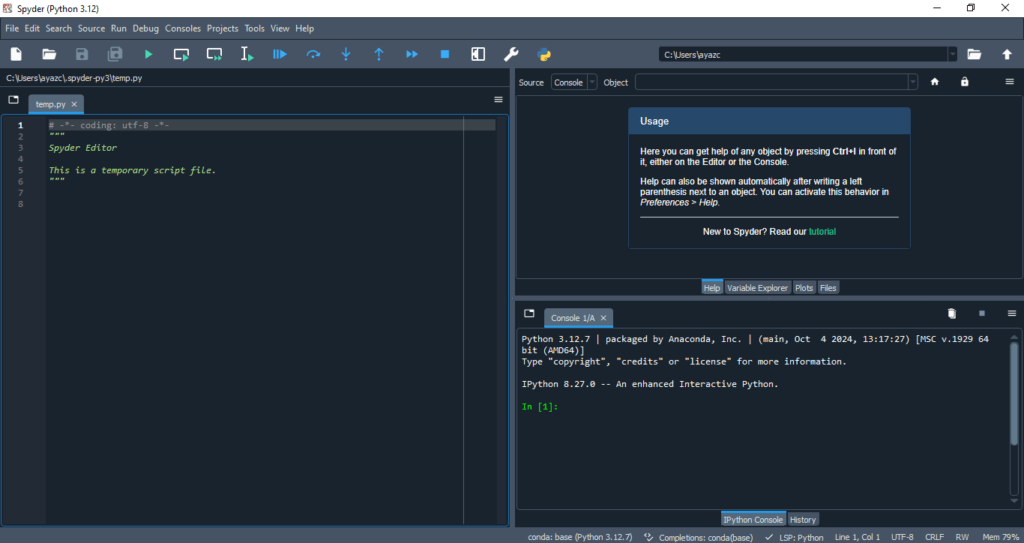
- Type the following code in the editor:
print("Hello, World!")
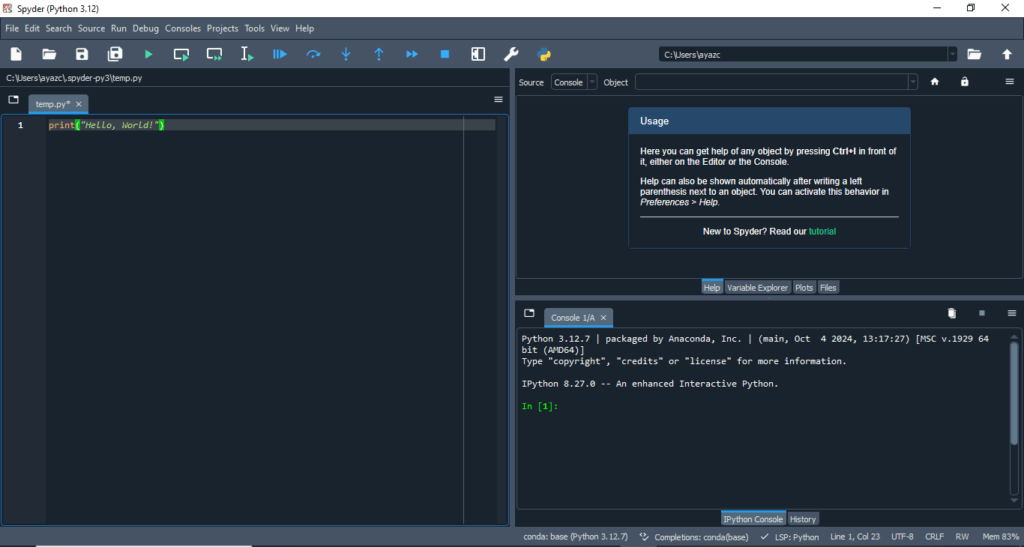
- Save the file with a
.py
extension, such ashello_world.py
.
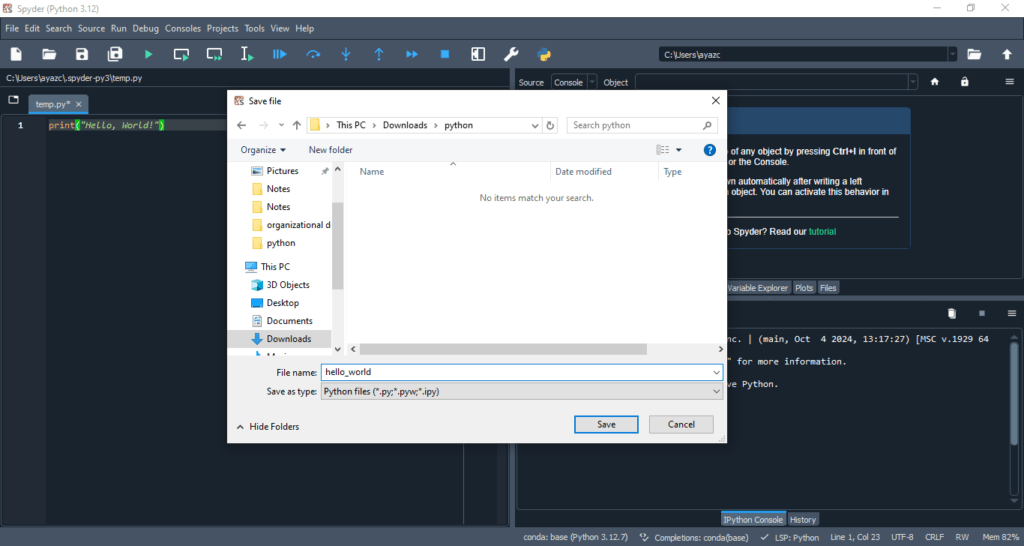
Run the Program
- In the IPython Console at the bottom of the window, you’ll see the output of your program.
- To run the program, click the Run button (a green triangle) on the toolbar or press F5.
Output
In the IPython Console, you will see:
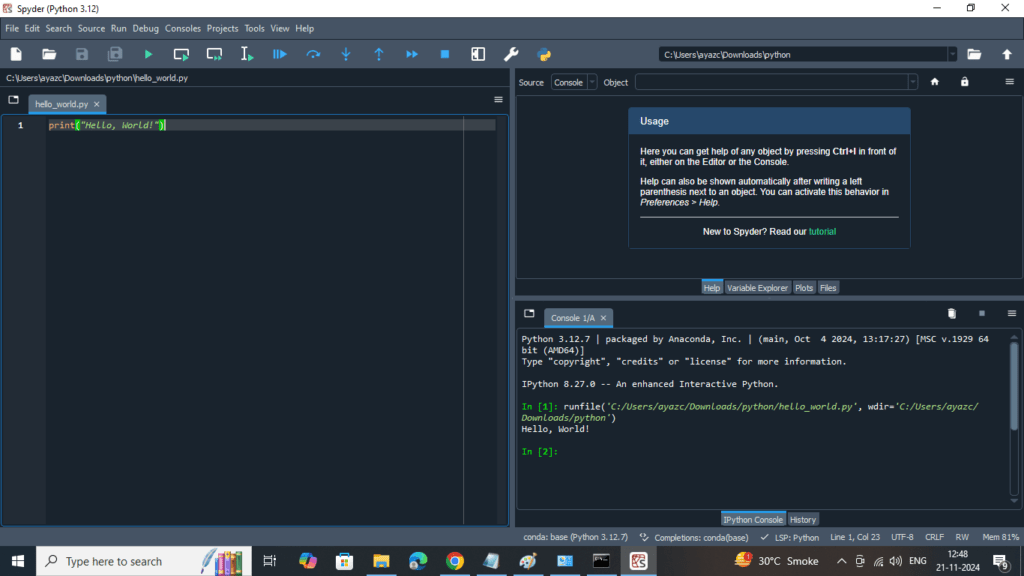
Why Use Spyder? Spyder is tailored for data science and scientific computing. It offers features like variable exploration and debugging, making it ideal for anyone doing data-intensive work.
➤ Running “Hello, World!” in Atom
Atom is a flexible, open-source text editor with support for Python through plugins.
Download and Install Atom
- Visit the official Atom website.
https://github.com/atom/atom/releases/tag/v1.60.0 - Download the installer compatible with your operating system (Windows/Mac/Linux).
- Once the download is complete, run the installer and follow the on-screen instructions to install Atom on your computer.

Open Atom
- Launch Atom from your Start menu or Applications folder.
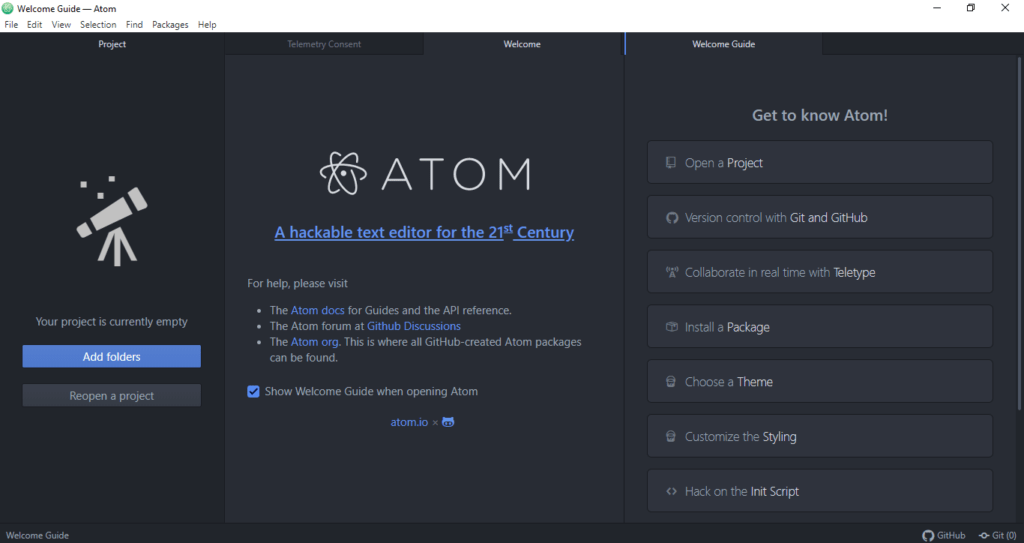
- If it’s your first time using Atom, close the “Welcome Guide” to access the main editor interface.
Install the Script Package
The Script package allows you to run scripts directly within Atom. Here’s how to set it up:
Download the Script Package from GitHub:
- Go to the Script GitHub Repository.
- Click Code > Download ZIP to download the package.
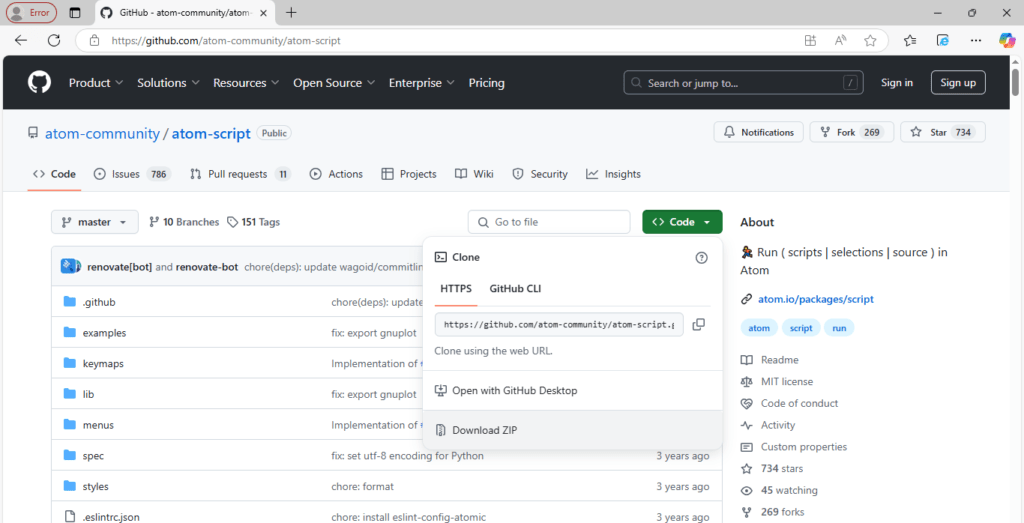
- Extract the ZIP file to a local folder.
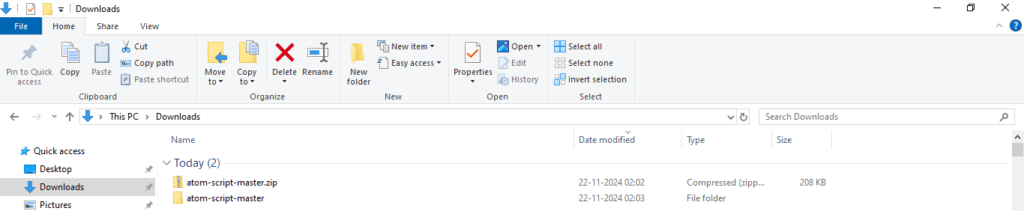
- Copy the extracted folder into the
packages
directory inside the.atom
folder:
On Windows:C:\Users\<your-username>\.atom\packages
On macOS/Linux:~/.atom/packages
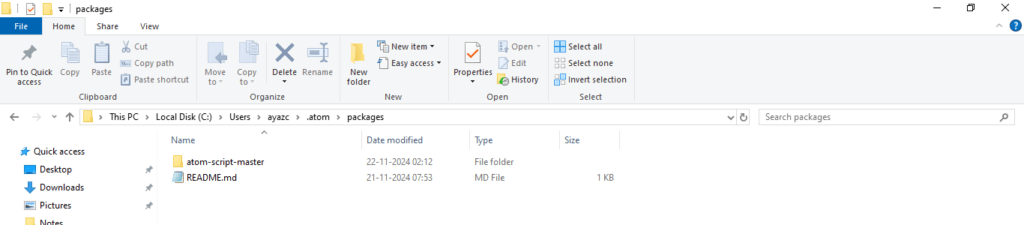
Fix Missing Dependencies (If Needed)
If you encounter an error (e.g., “Cannot find module ‘temp'”), follow these steps:
Install Node.js and npm:
- Download Node.js from https://nodejs.org.
- Follow the installer instructions.
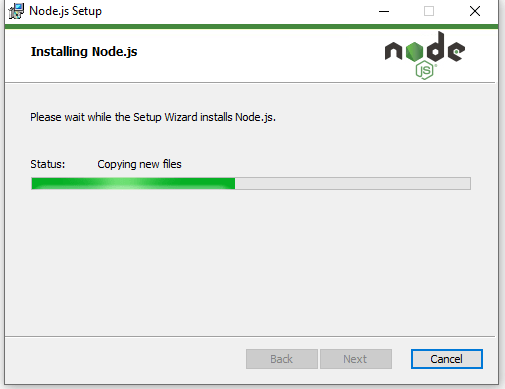
- Verify installation:
node -v
npm -v
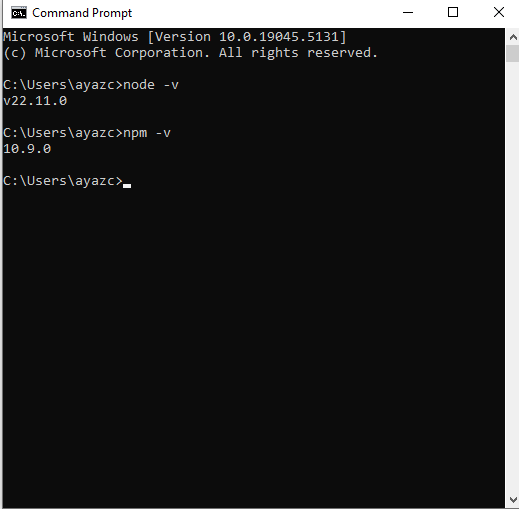
Manually Install the Missing Dependency:
- Open a terminal or command prompt.
- Navigate to the Script package directory:
cd C:\Users\<your-username>\.atom\packages\atom-script-master
- Install the
temp
module:npm install temp
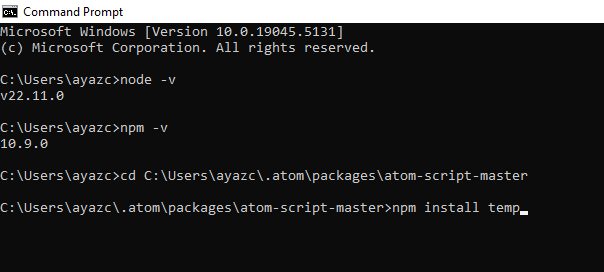
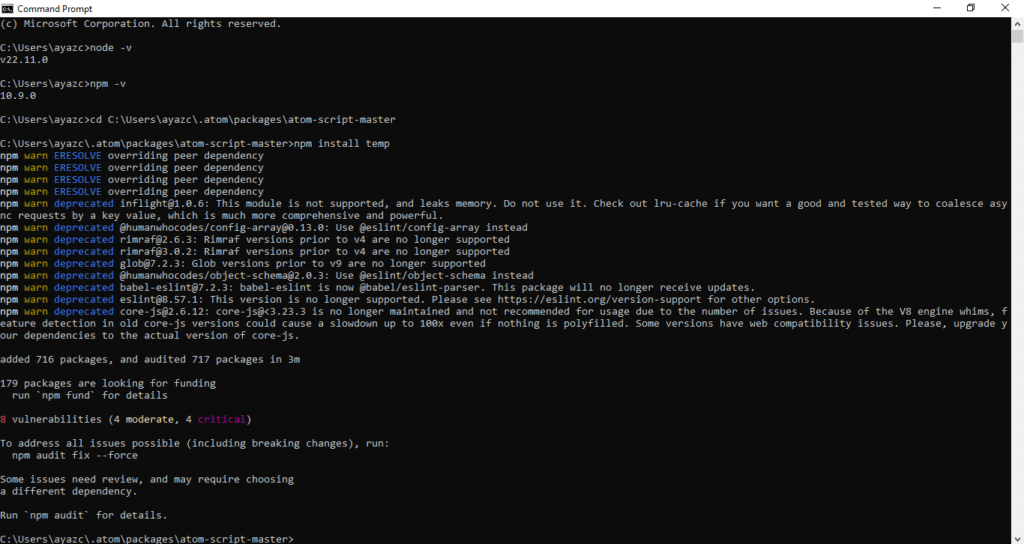
- Restart Atom and try running your script again.
Create a Python File
- In Atom, click File > New File to create a new file.
- Save the file by clicking File > Save As, and give it a name like
hello_world.py
. Make sure the file extension is.py
to indicate it’s a Python file.
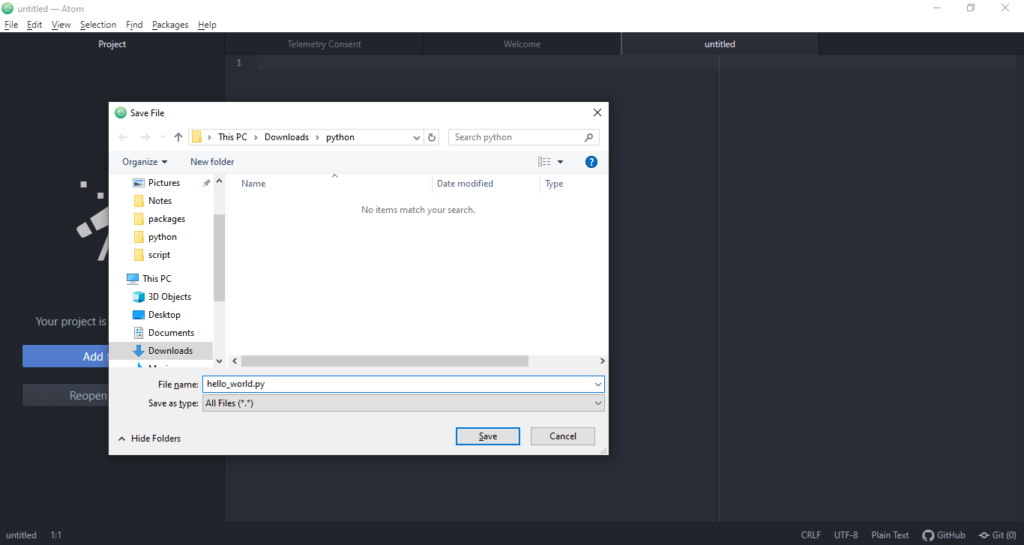
Write the “Hello, World!” Program
- Type the following code into the new file:
print("Hello, World!")
- Save the file by pressing
Ctrl + S
(Windows) orCmd + S
(macOS).
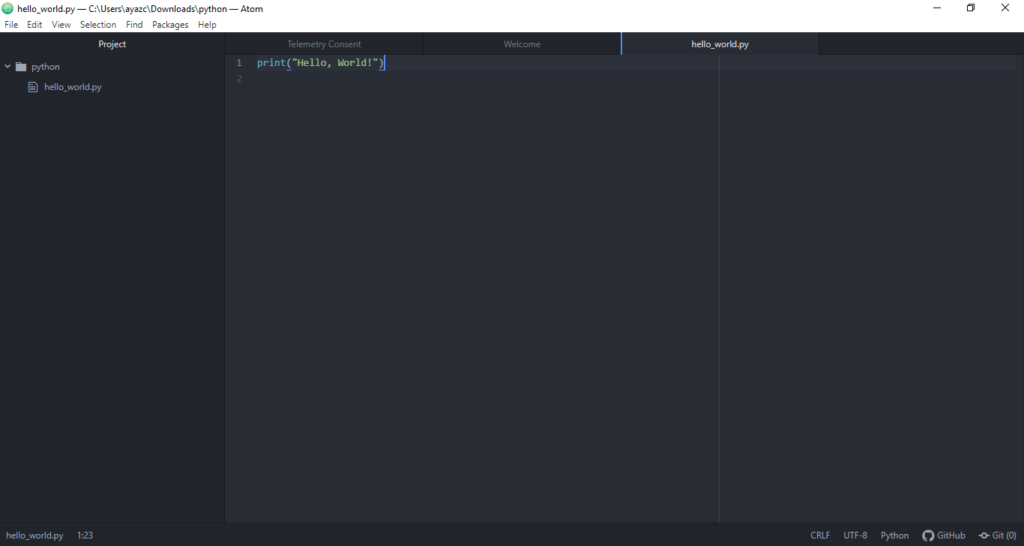
Run the Program in Atom
- Select Script > Run Script or press the shortcut (
Shift+Ctrl+B
on Windows/Linux,Shift+Cmd+B
on macOS).
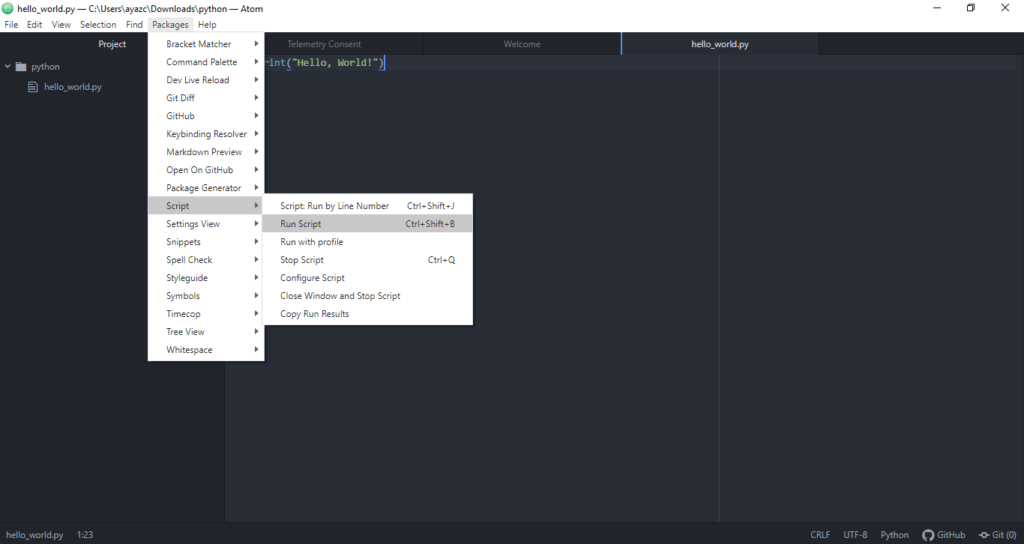
Output
The output will appear in the bottom panel of Atom.
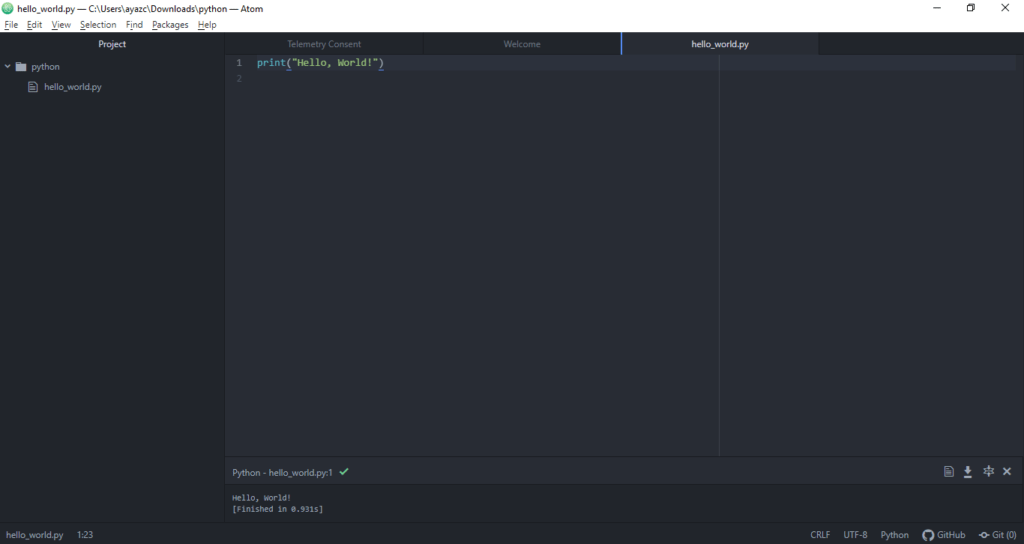
Why Use Atom? Atom is highly customizable with a wide range of packages. It’s lightweight, but its plugin system makes it highly extendable.
➤ Running “Hello, World!” in Sublime Text
Sublime Text is a fast and minimalist text editor that can be configured for Python development.
Download Sublime Text:
- Go to the official Sublime Text website and download the appropriate version for your operating system (Windows, macOS, or Linux).
Install Sublime Text:
- Windows: Run the
.exe
installer you downloaded and follow the on-screen instructions. - macOS: Open the
.dmg
file and drag Sublime Text to your Applications folder. - Linux: You can install Sublime Text using a package manager like
apt
(Ubuntu),dnf
(Fedora), oryum
(CentOS).
Install the Python Package in Sublime Text
Although Sublime Text supports many programming languages out of the box, you need to install a Python package to ensure that Sublime Text can run Python code correctly.
Open Sublime Text: Once the installation is complete, launch Sublime Text.
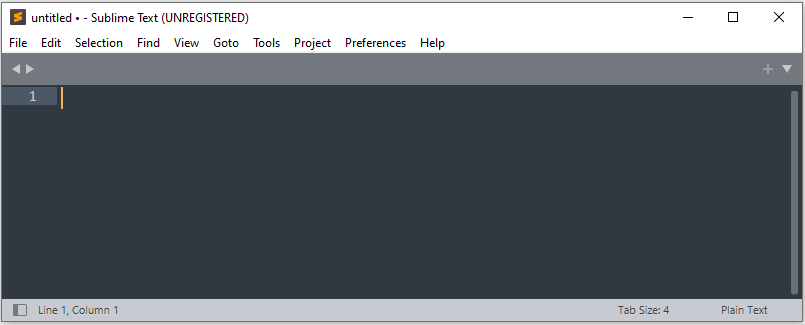
Install Package Control (if not already installed):
- Open the Command Palette by pressing Ctrl + Shift + P (Windows/Linux) or Cmd + Shift + P (macOS).
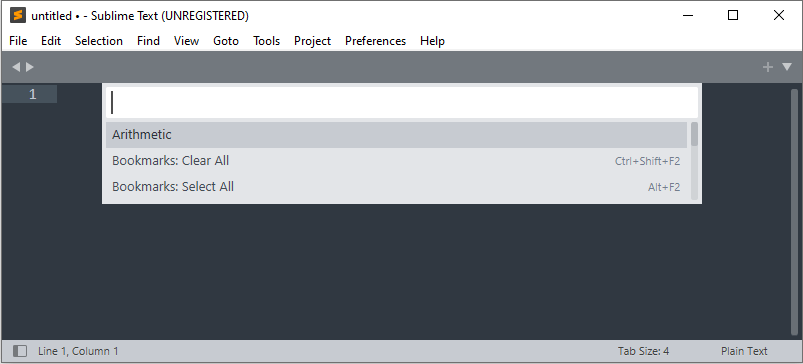
- Type
Install Package Control
and hit Enter. This will install the package manager for Sublime Text.
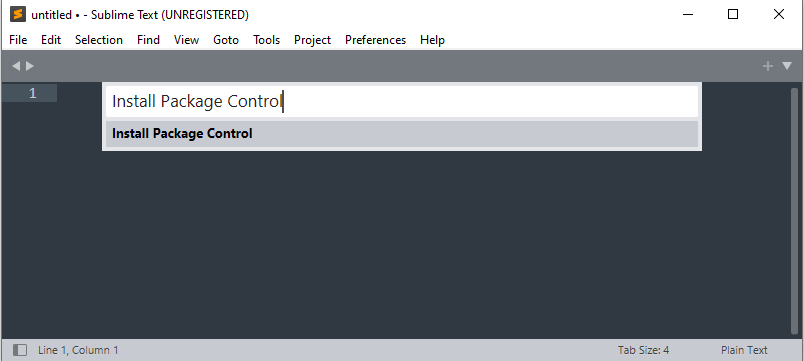
Install the Python Package:
- Once Package Control is installed, open the Command Palette again.Type
Package Control: Install Package
and hit Enter.
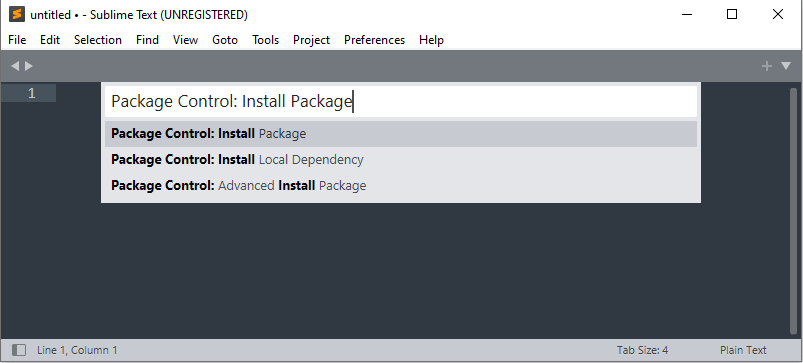
- In the search bar, type
Python
and select the Python package to install it.
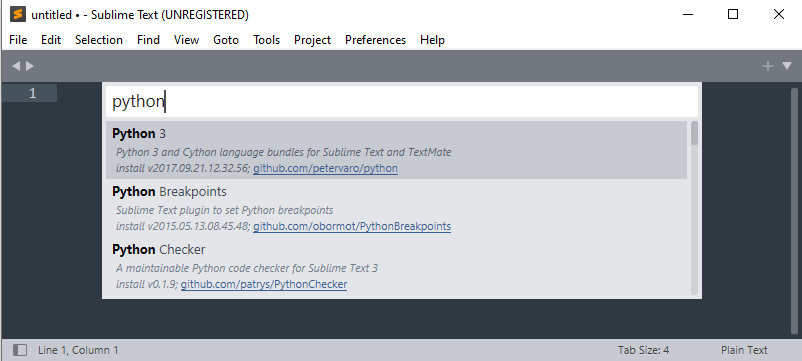
This step will enable Python-specific features such as syntax highlighting and running Python scripts directly from Sublime Text.
Create a New File:
- Open a new file by going to File > New File or pressing Ctrl + N (Windows/Linux) or Cmd + N (macOS).
- In the new file, type the following Python code:
print("Hello, World!")
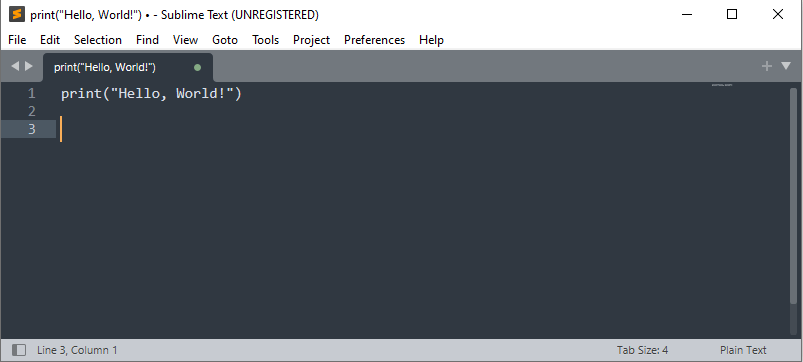
- Save the file with a
.py
extension (e.g.,hello_world.py
) by going to File > Save As or pressing Ctrl + S (Windows/Linux) or Cmd + S (macOS). Choose a location on your computer to save the file.
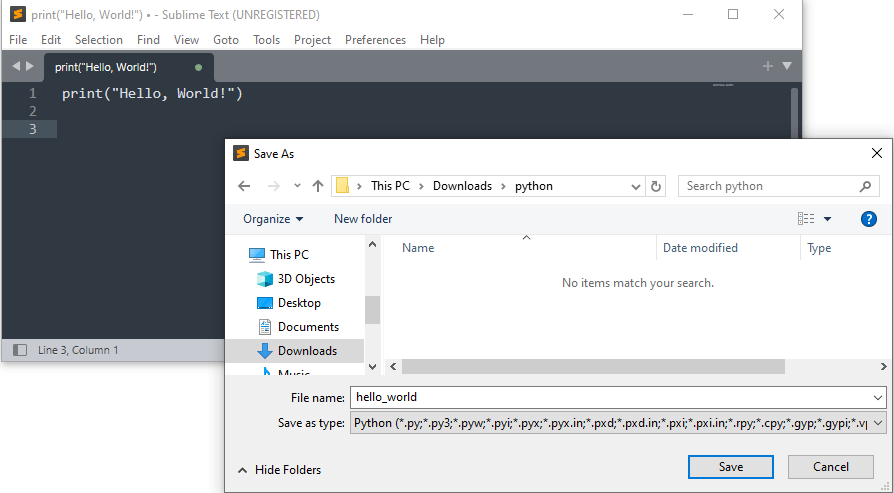
Run the Python Script
To run Python code directly from Sublime Text, you can use the Build System feature. Here’s how you do it:
Select Python Build System:
- Go to Tools > Build System > Python. This will tell Sublime Text to use Python to execute your code.
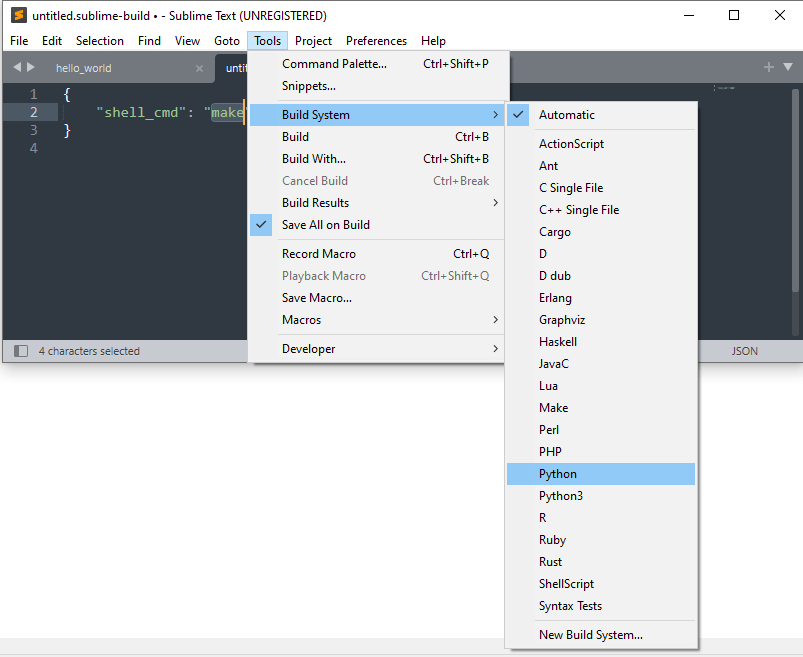
Run the Script:
- Press Ctrl + B (Windows/Linux) or Cmd + B (macOS) to run the script.
- Alternatively, you can also go to Tools > Build from the top menu.
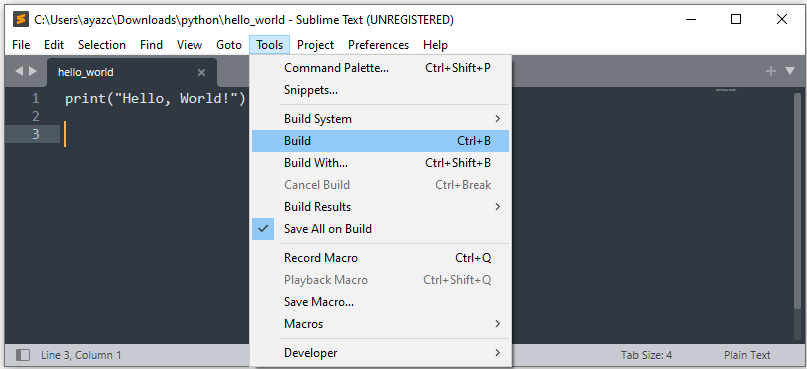
Output
After running the script, the output will appear in the Build Output panel at the bottom of the Sublime Text window.
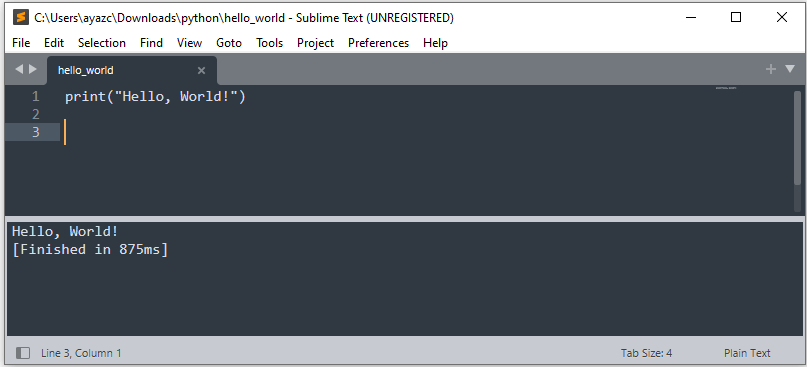
Why Use Sublime Text? Sublime Text is perfect for developers who like fast, distraction-free coding with a sleek interface. It can be extended through packages to suit your needs.
➤ Running “Hello, World!” in PyDev
PyDev is a plugin for Eclipse that allows you to write and run Python code inside the Eclipse IDE.
Download Eclipse IDE:
- Visit the Eclipse download page.
- Download the Eclipse Installer.
- Run the installer and follow the on-screen instructions to install Eclipse Installer on your computer.
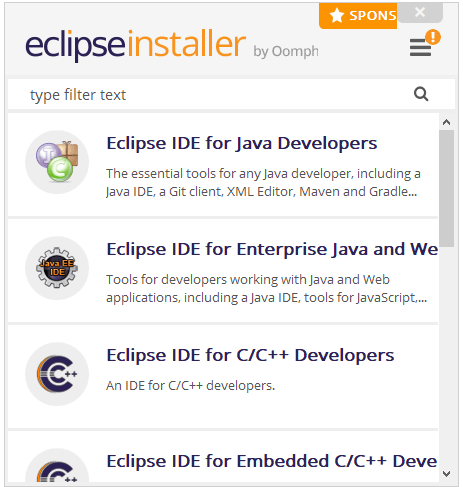
- Install the version suitable for your system (Eclipse IDE for Java Developers is a good choice).
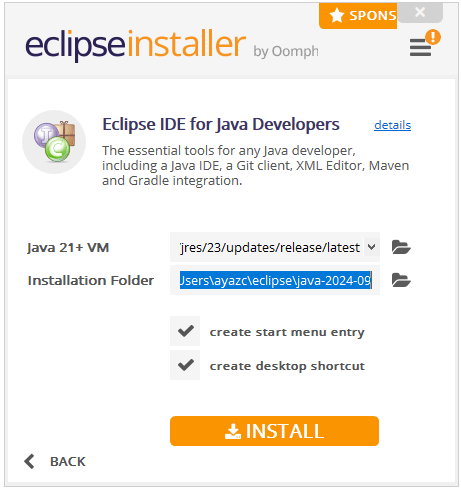
Install Eclipse:
- Run the installer and follow the on-screen instructions to install Eclipse on your computer.
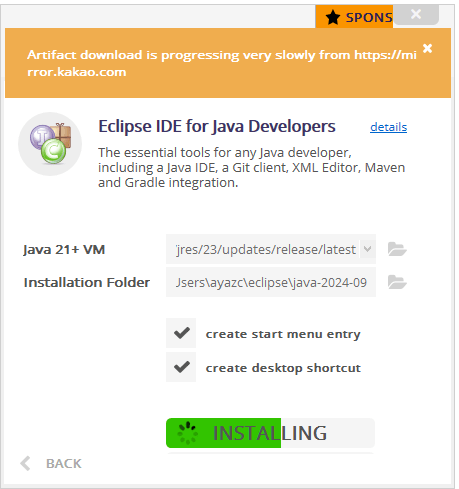
- Once installed, launch Eclipse.
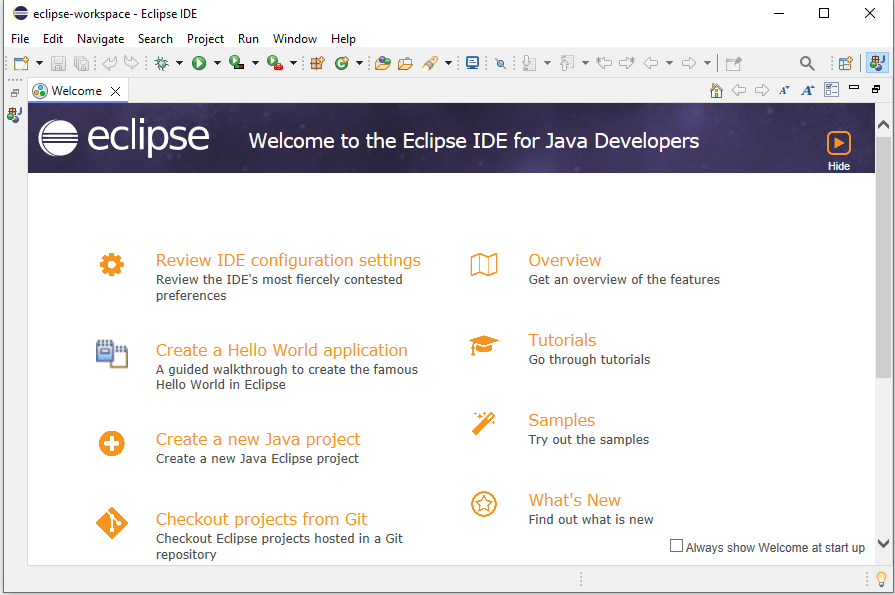
Install PyDev:
- Go to Help in the top menu bar and select Eclipse Marketplace….
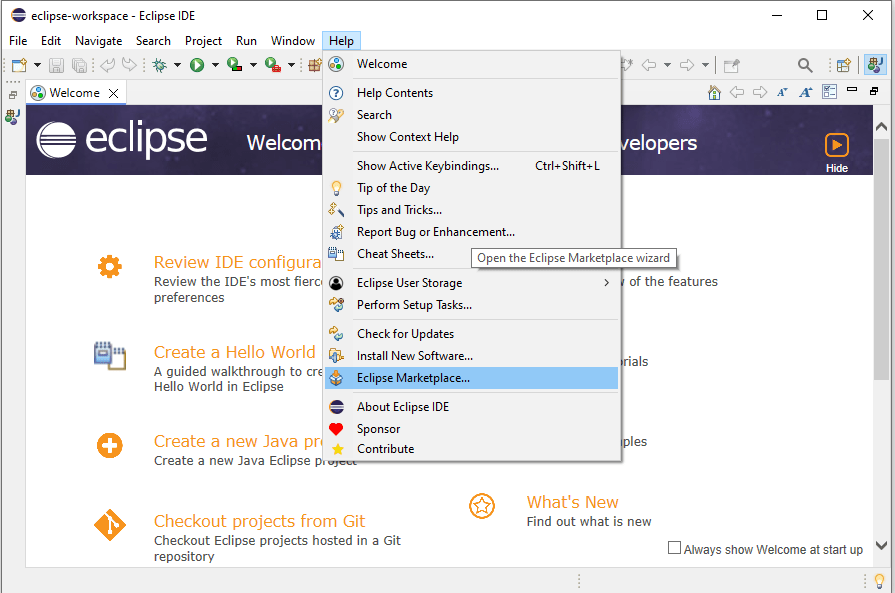
- In the Eclipse Marketplace, search for PyDev using the search bar.
Click Go, and you should see PyDev for Eclipse in the list of available plugins.
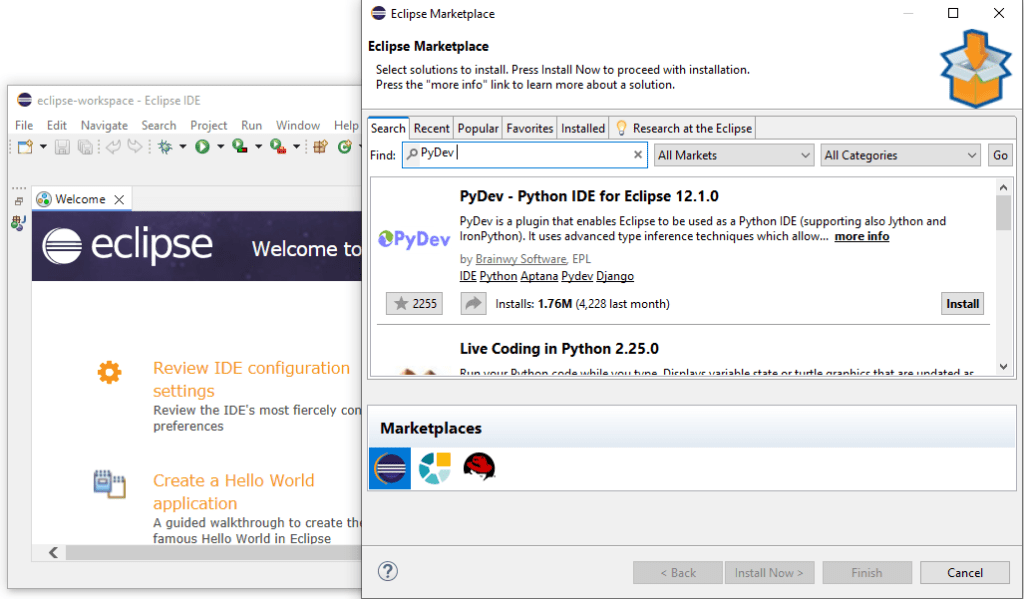
- Click the Install button next to PyDev for Eclipse.Follow the installation prompts, and restart Eclipse when asked to complete the installation.
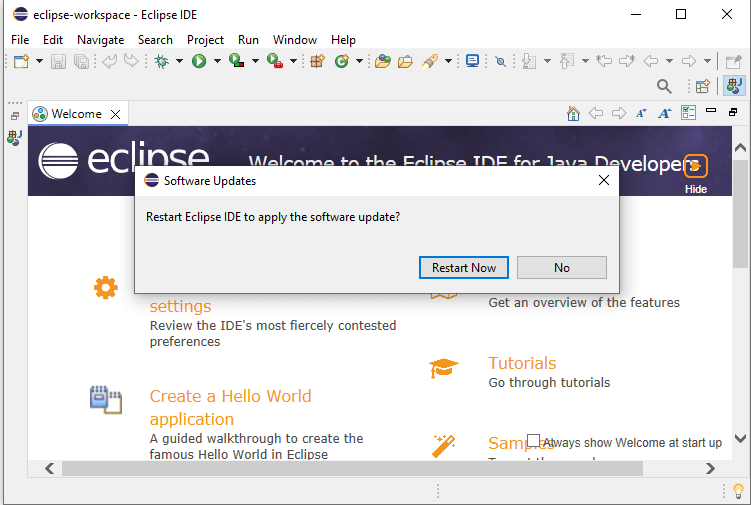
Set Up Python Interpreter in Eclipse
- Before you can run Python programs, you need to configure the Python interpreter in Eclipse.
Steps to Configure Python Interpreter:
- In Eclipse, go to Window in the top menu bar, and select Preferences.
- In the Preferences window, expand the PyDev section on the left-hand side.
- Select Interpreters > Python Interpreter.
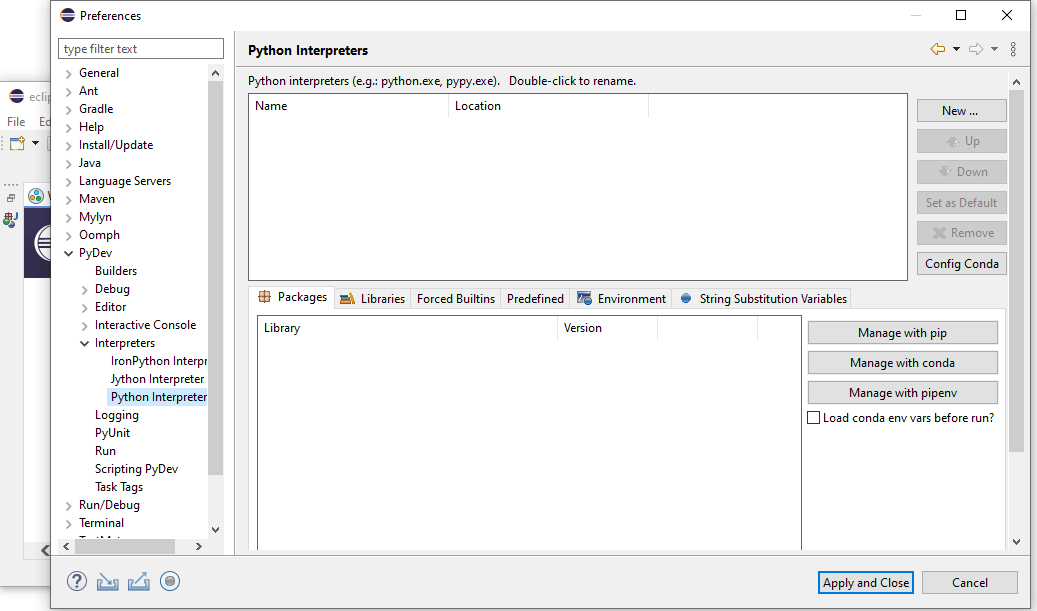
- Click the New… button to add a Python interpreter.
- Navigate to the location where Python is installed on your system and select the Python executable (e.g.,
python.exe
on Windows orpython3
on macOS/Linux).
- Click OK to confirm, then click
Apply and Close
and close the Preferences window.
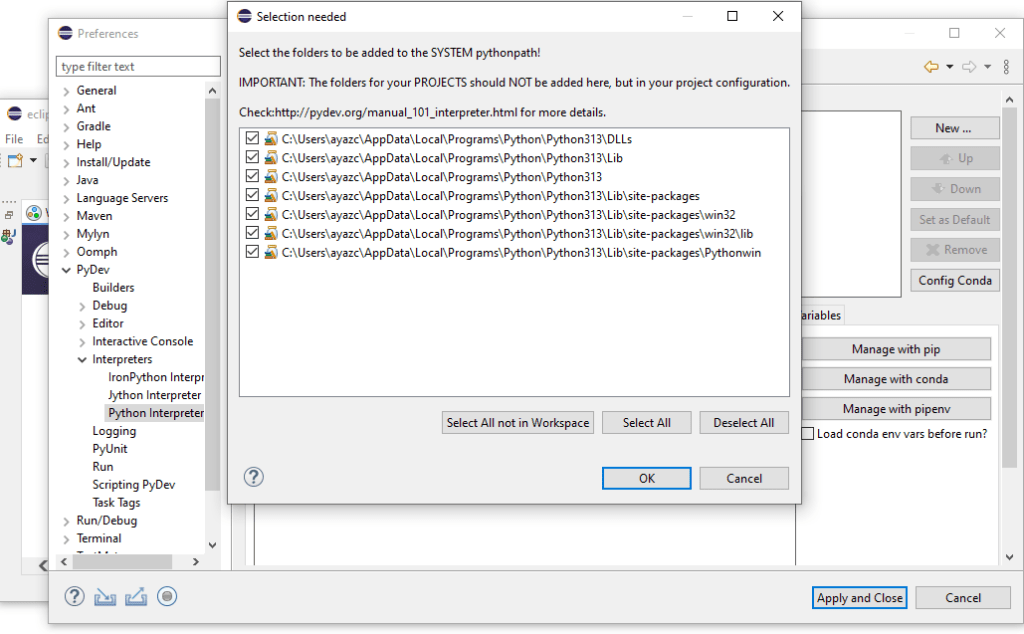
Create a New Python Project:
- In Eclipse, go to File > New > Project….
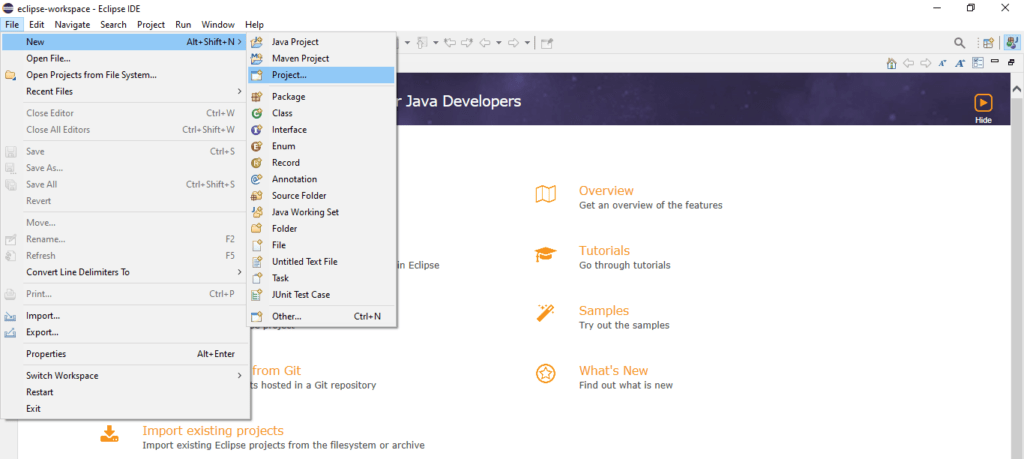
- Under the PyDev section, select PyDev Project and click Next.
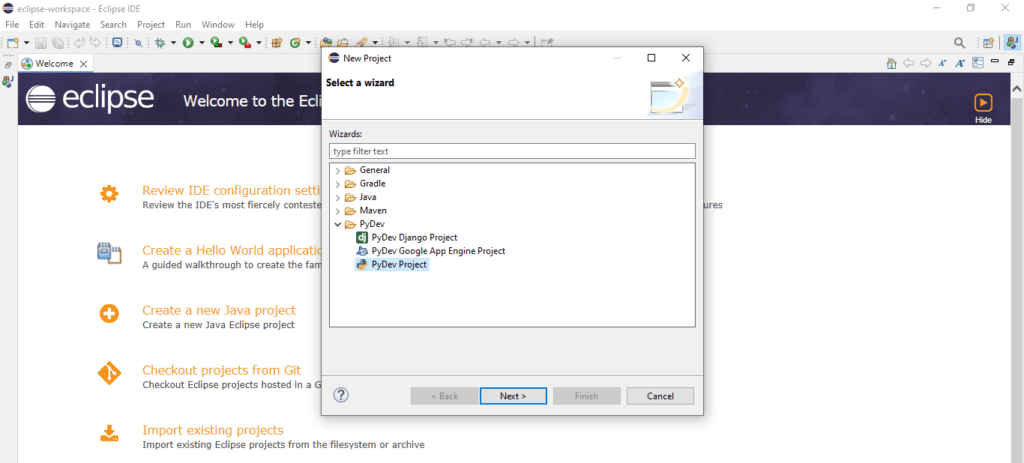
- Enter a name for your project (e.g.,
HelloWorldProject
). - Ensure that the Python interpreter is set to the interpreter you just configured.
- Click Finish to create the project.
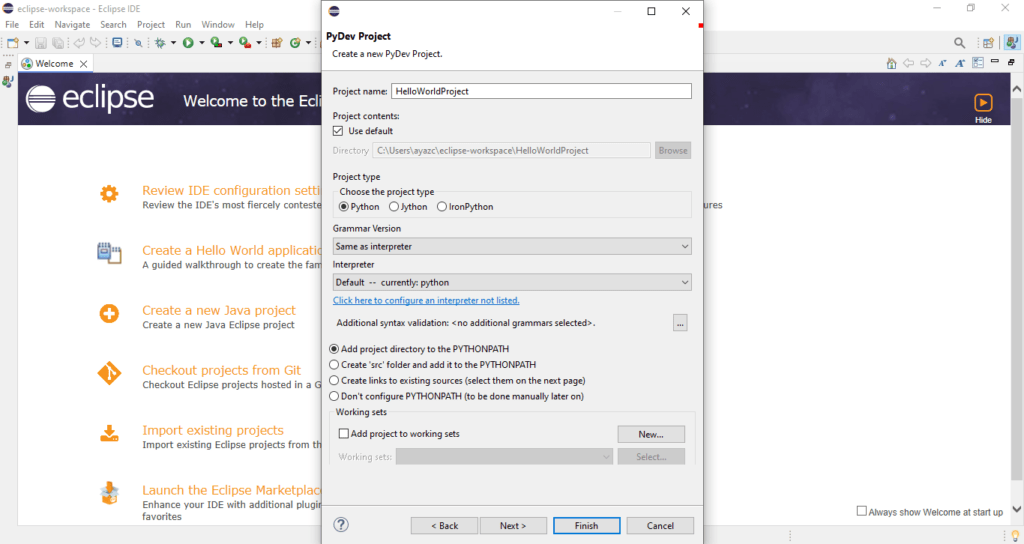
Create a Python File:
- Right-click on your project name (e.g.,
HelloWorldProject
) in the Project Explorer on the left-hand side. - Select New > File.
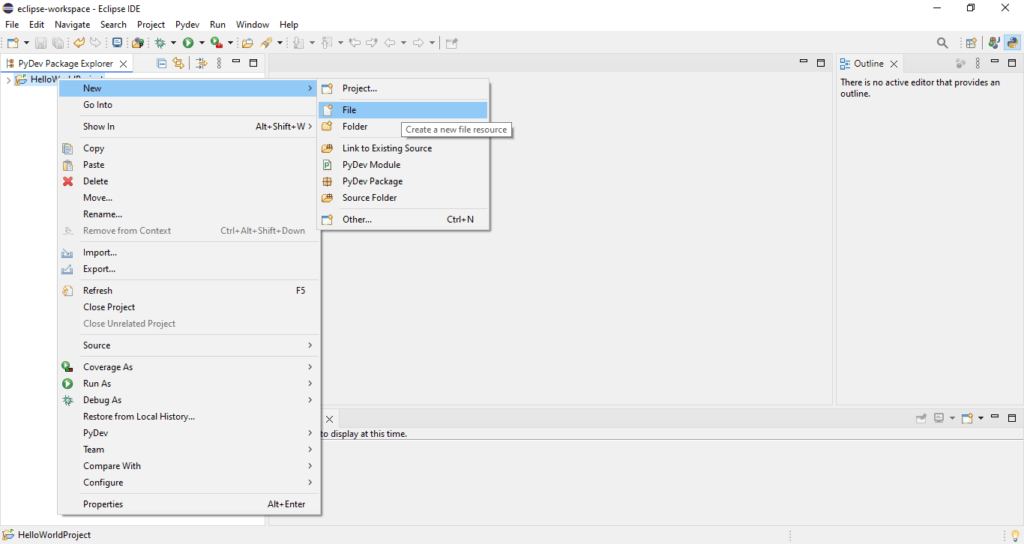
- Enter the file name as
hello_world.py
(or any name you prefer, but with a.py
extension). - Click Finish.
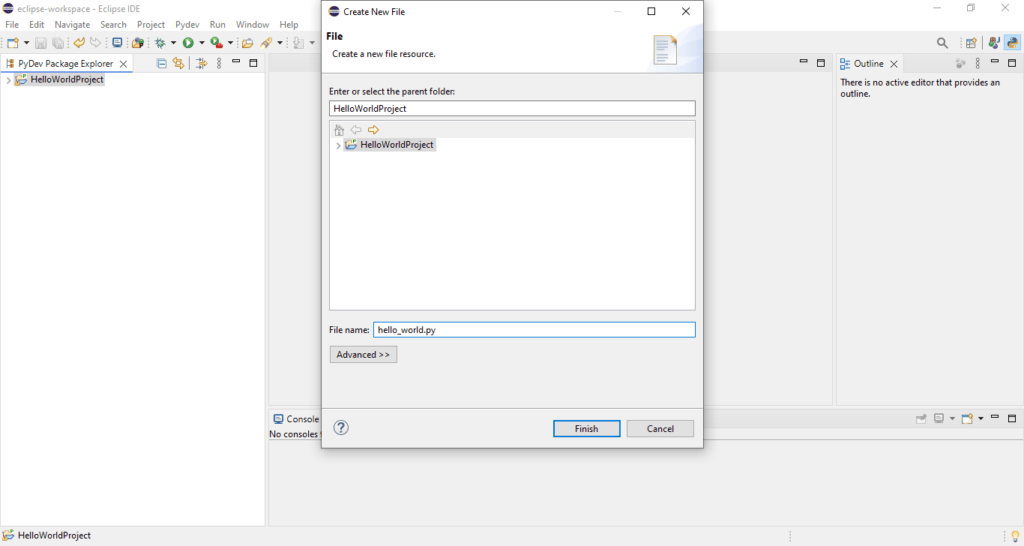
Write Your Python Code
Write the Code:
- In the editor window, type the following code:
print("Hello, World!")
- Save the file by clicking File > Save or pressing Ctrl + S.
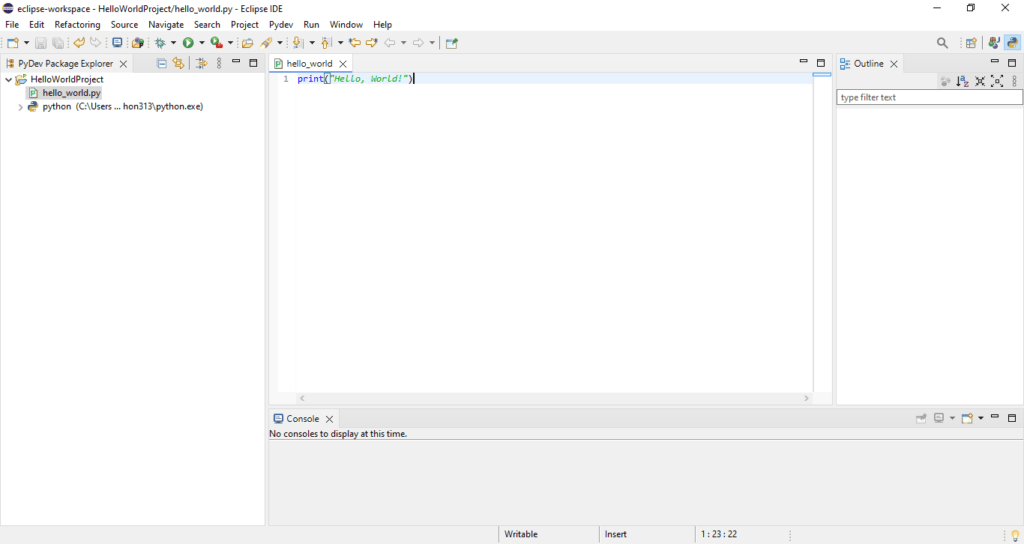
Output
Right-click on your Python file (hello_world.py
) in the Project Explorer.
Select Run As > Python Run from the context menu.
After running the program, the output will appear in the Console window at the bottom of the Eclipse IDE.
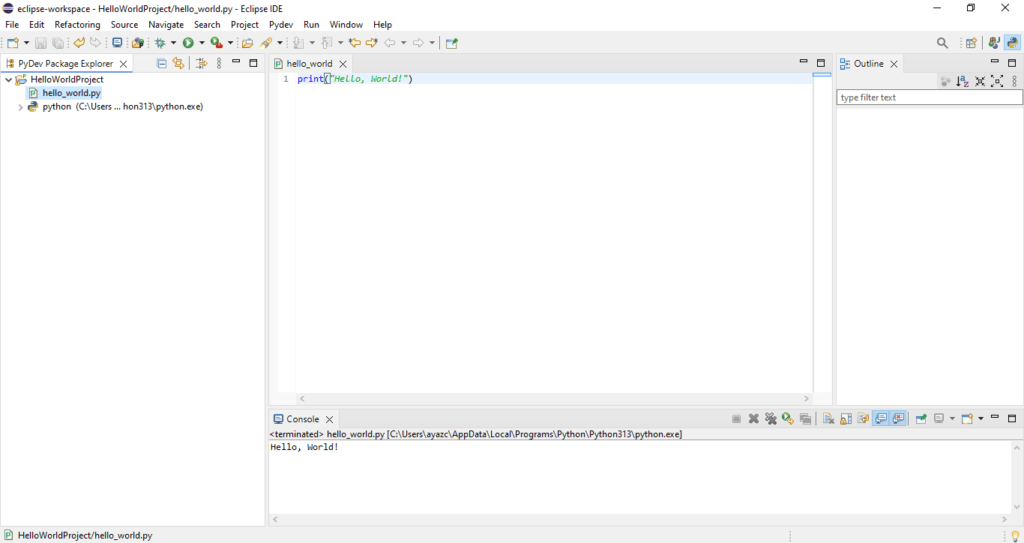
Why Use PyDev? If you’re already using Eclipse for Java or other programming languages, PyDev integrates well with your existing setup.
➤ Running “Hello, World!” in Vim
Vim is a terminal-based text editor that’s highly customizable, but it has a steep learning curve.
Open Command Prompt:
- Press Win + R to open the Run dialog box.
- Type
cmd
and press Enter to open the Command Prompt.
Check if Vim is already installed by typing vim --version
.
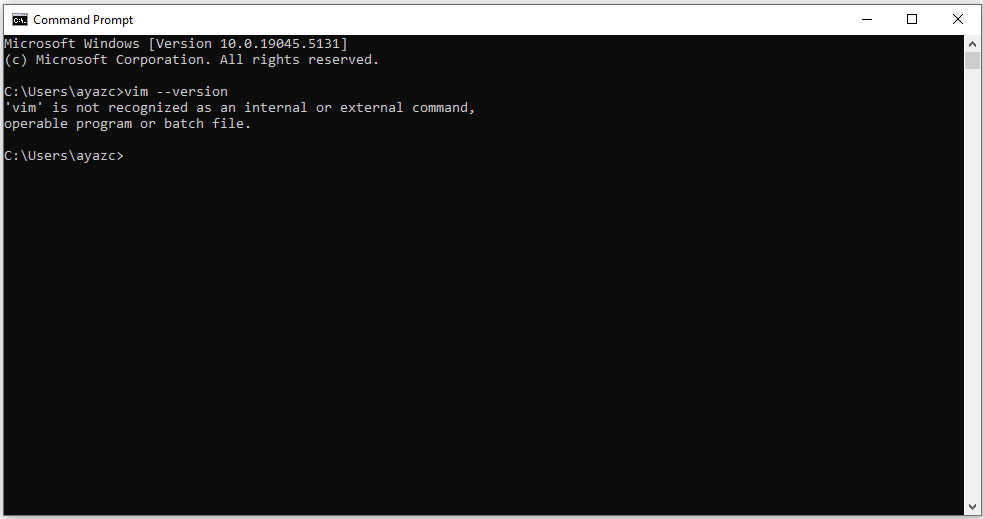
If Vim is not installed, download it by following these steps:
- Go to the official Vim website and download the installer for Windows.
- Run the installer and follow the on-screen instructions to install Vim.
- After installation, verify by typing
vim --version
.
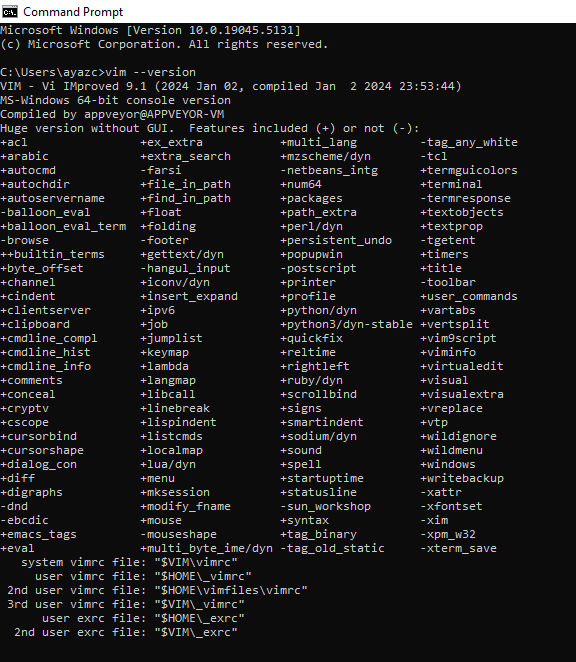
Navigate to the folder where you want to save your file using the cd
command.
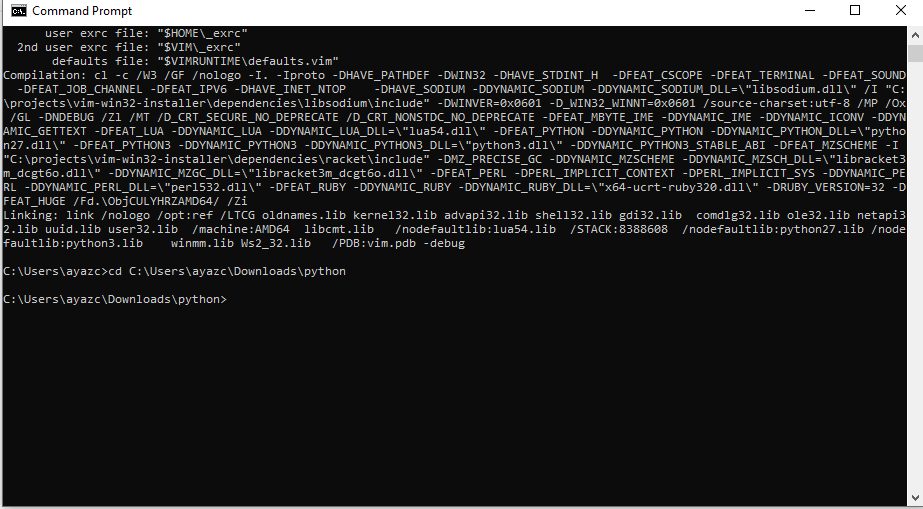
Open Vim and create a new file called hello_world.py
by typing vim hello_world.py
.
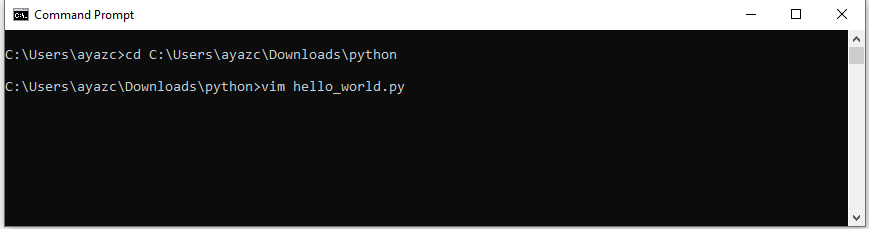
Once Vim opens, press i to enter Insert Mode.
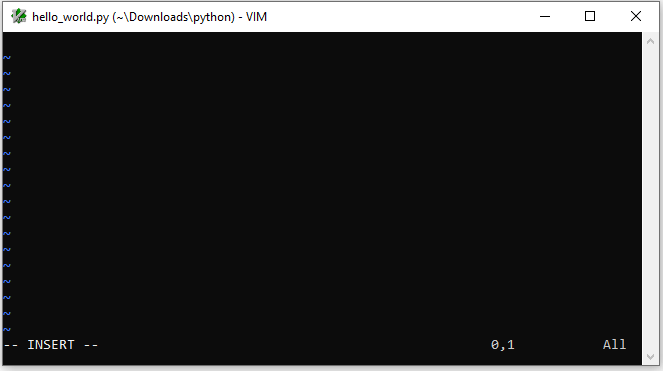
You can now type your Python code.
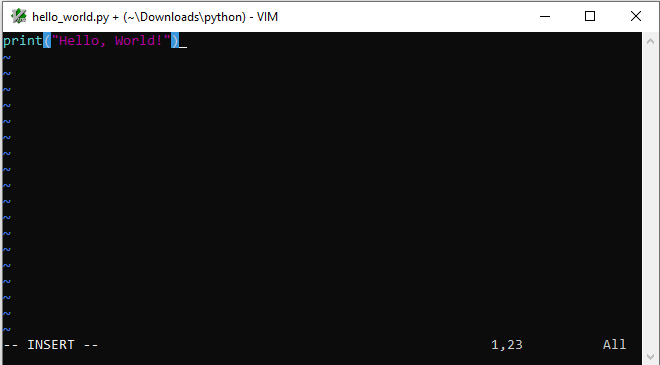
After writing the code, press Esc to exit Insert Mode. Then save and exit by typing :wq
.
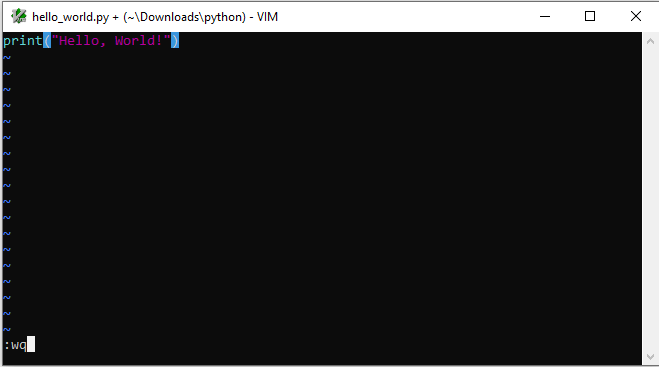
Back in the command prompt, run the Python file using the following command python hello_world.py
.
Output
Press Enter to see the output.
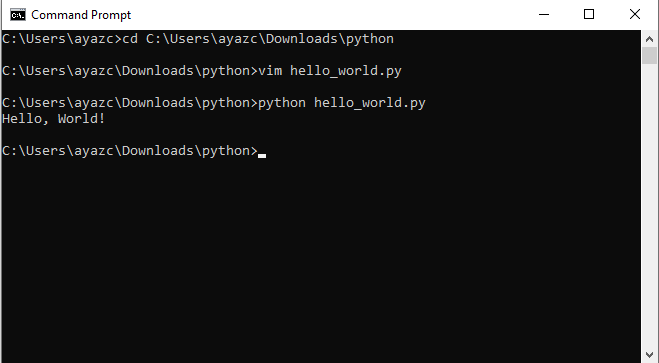
Why Use Vim? Vim is loved by experienced developers for its speed and keyboard shortcuts. It’s great for working in the terminal and editing code without leaving the console.
Tips
- If you’re new to Vim, practice basic commands like
i
(insert),Esc
(exit insert),:wq
(save and quit), and:q!
(quit without saving). - Customize Vim for Python development by adding plugins like YouCompleteMe or ALE for linting and autocompletion.
➤ Running “Hello, World!” in iPython
IPython is an enhanced interactive shell that offers more features than the regular Python shell.
Open Command Prompt:
- Press Win + R to open the Run dialog box.
- Type
cmd
and press Enter to open the Command Prompt.
Install IPython (if not installed):
Type the command pip install ipython
in the Command Prompt.
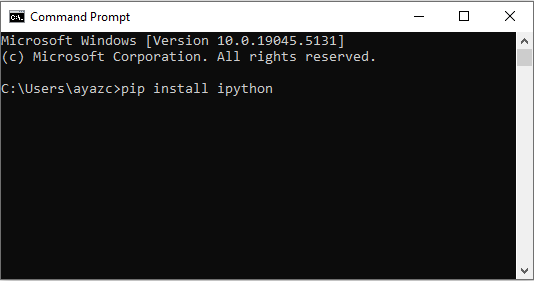
Press Enter and Wait for the installation to complete. You’ll see a message like:Successfully installed ipython-X.X.X
.
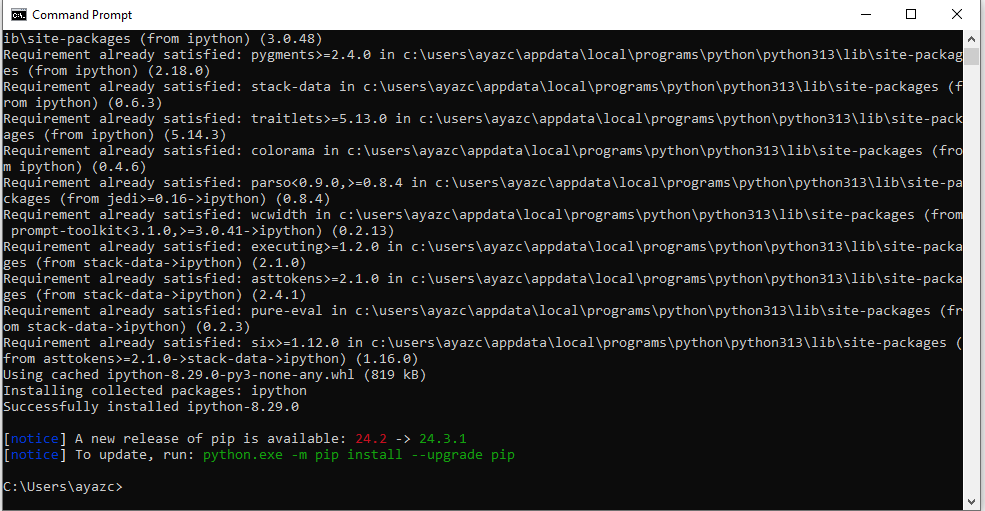
Launch IPython in the terminal by typing ipython
.
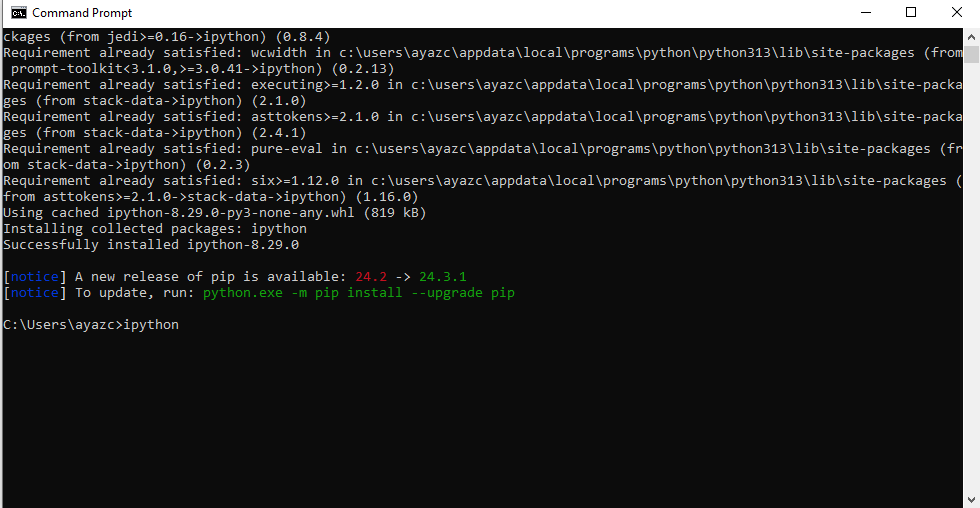
Type print("Hello, World!")
.
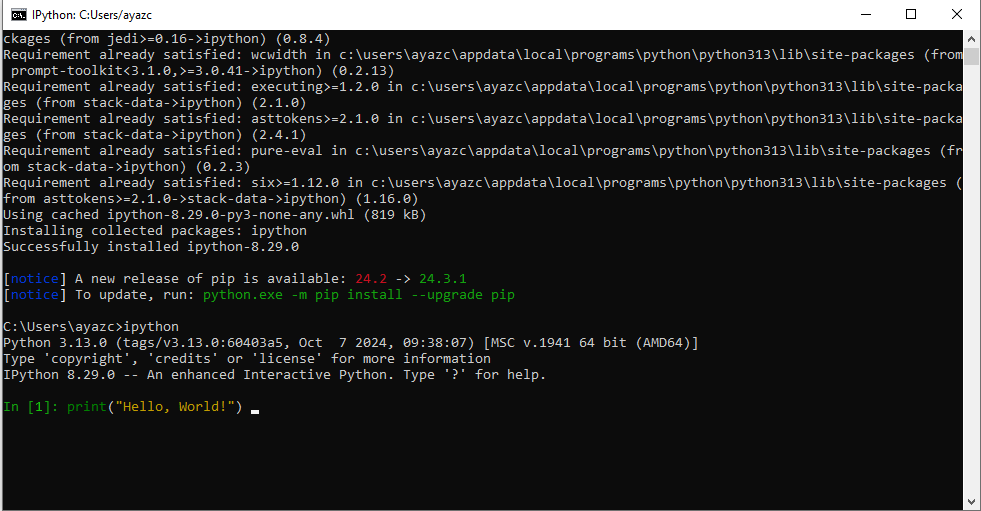
Output
Press Enter to see the output.
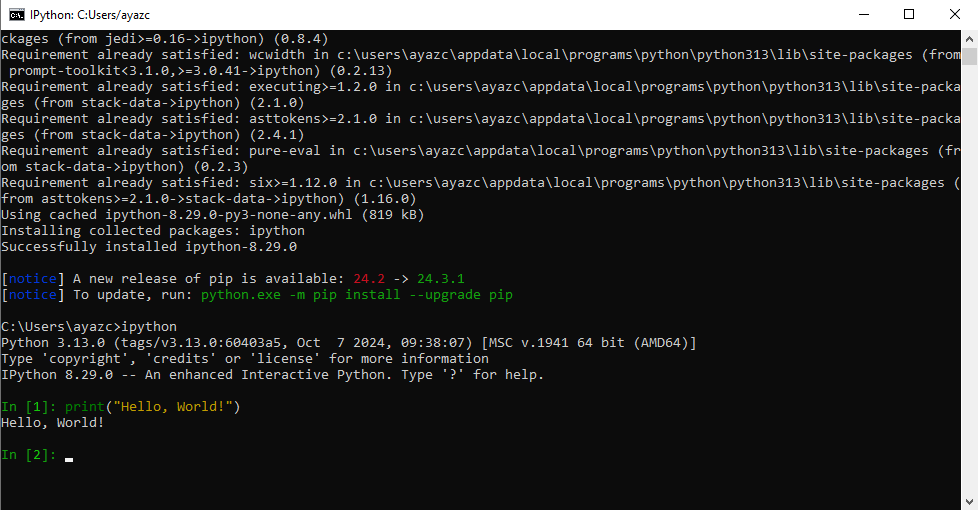
Why Use IPython? IPython is perfect for quick experimentation and interactive coding. It’s commonly used in data science and research environments.
❉ Conclusion
You’ve successfully written and executed your first “Hello, World!” program across 14 different IDEs and environments. Each IDE has its strengths, and the best one depends on your specific needs:
- For beginners, IDLE, Thonny, and PyCharm are excellent choices.
- If you’re into data science, Jupyter and Spyder will serve you well.
- For a lightweight and customizable experience, VS Code, Atom, and Sublime Text are great options.
- If you prefer minimal setups, Notepad++ and Command Prompt/Terminal provide straightforward environments to run Python programs effectively.
Remember, the goal is to experiment with different tools to find the one that suits you best. No matter which environment you use, the core Python syntax remains the same. The more you practice, the better you’ll get at using these environments efficiently. Happy coding!