Random Password Generator with Python
Building a Robust Random Password Generator and Password Strength Checker
In today’s digital world, security is paramount. One of the most critical aspects of security is ensuring strong and unique passwords. In this post, we’ll walk through creating a Python project that can generate random passwords, assess their strength, and even save them securely to a file. This project involves various Python concepts, including string manipulation, file I/O operations, and random number generation.
Project Overview
We will build a Random Password Generator that allows us to:
- Generate a password with customizable length and character set (uppercase, lowercase, digits, special characters).
- Check the strength of the password (Weak, Medium, Strong).
- Save the generated password to a file for future reference.
Flow of the Program
The flow of the program is as follows:
- Start: The program begins by displaying the prompt for the password length and other preferences.
- Password Generation: The user inputs their desired password length and whether they want special characters in the password.
- Password Strength Check: The program generates a password and checks its strength.
- Display and Save: The password is displayed along with its strength. The user is asked if they want to save the password to a file.
- End: If the user accepts the password, the program saves it to a file and ends. If not, the program loops back and asks for a new password until the user is satisfied.
Breaking Down the Code
Let’s take a deep dive into each section of the code to understand how everything works and how you can apply similar concepts to your own projects.
1. Importing Libraries
import random
import string
import os
We begin by importing the necessary libraries:
random
: This module helps generate random choices from a list, making it perfect for password creation.string
: This module provides easy access to common sets of characters, such as lowercase, uppercase, digits, and punctuation.os
: This library is used to interact with the operating system, primarily for file handling.
2. Password Generation Function
def generate_password(length, use_special=True):
"""
Generate a random password based on the specified length and character preferences.
"""
if length < 4:
raise ValueError("Password length must be at least 4 characters.")
# Define character pools
lower_case = string.ascii_lowercase
upper_case = string.ascii_uppercase
digits = string.digits
special_characters = string.punctuation if use_special else ""
# Combine character pools
all_characters = lower_case + upper_case + digits + special_characters
# Ensure at least one character from each mandatory pool
password = [
random.choice(lower_case),
random.choice(upper_case),
random.choice(digits),
]
if use_special:
password.append(random.choice(special_characters))
# Fill the rest of the password
for _ in range(length - len(password)):
password.append(random.choice(all_characters))
# Shuffle and return the password
random.shuffle(password)
return "".join(password)
- Input Parameters:
length
: The length of the password to be generated. The function checks if the length is at least 4 characters.use_special
: A boolean flag that determines whether special characters should be included.
- Password Construction: The password is constructed by:
- First selecting one random character from each required category: lowercase, uppercase, and digits. If special characters are allowed, one is selected as well.
- The rest of the password is then randomly filled with characters from the combined pool of lowercase, uppercase, digits, and special characters.
- Shuffling: To ensure randomness, the order of characters is shuffled before returning the password.
3. Password Strength Check Function
def check_password_strength(password):
"""
Assess the strength of a password.
"""
length = len(password)
has_lower = any(char.islower() for char in password)
has_upper = any(char.isupper() for char in password)
has_digit = any(char.isdigit() for char in password)
has_special = any(char in string.punctuation for char in password)
if length >= 12 and has_lower and has_upper and has_digit and has_special:
return "Strong"
elif length >= 8 and has_lower and has_upper and (has_digit or has_special):
return "Medium"
else:
return "Weak"
This function evaluates the strength of a given password:
- Length: Checks if the password is at least 12 characters for a strong password or 8 characters for a medium password.
- Character Categories: It ensures that the password contains at least one lowercase letter, one uppercase letter, one digit, and optionally, one special character.
- Return Strength: The function returns "Strong", "Medium", or "Weak" based on the criteria above.
4. Saving the Password to a File
def save_password_to_file(password, file_name="saved_passwords.txt"):
"""
Save the generated password to a file.
"""
with open(file_name, "a") as file:
file.write(password + "\n")
print(f"Password saved to {file_name}")
The function save_password_to_file
saves the generated password to a file:
- Appending to File: It opens a text file in append mode (
"a"
) and writes the password to it. If the file doesn't exist, it will be created.
- File Name: By default, passwords are saved in a file named
saved_passwords.txt
, but you can specify a different file name.
5. Main Program Flow
def main():
print("----------- Enhanced Random Password Generator -----------")
while True:
try:
length = int(input("Enter the length of the password (minimum 4): "))
if length < 4:
print("Password length must be at least 4 characters.")
continue
include_special = False # By default, don't include special characters for length of 4
if length > 4:
include_special = input("Include special characters? (yes/no): ").strip().lower() == "yes"
# Generate and display the password
password = generate_password(length, use_special=include_special)
print(f"Generated Password: {password}")
# Check password strength
strength = check_password_strength(password)
print(f"Password Strength: {strength}")
# Save password if confirmed
if input("Accept this password? (yes to confirm, no to regenerate): ").strip().lower() == "yes":
save_option = input("Save this password to a file? (yes/no): ").strip().lower()
if save_option == "yes":
save_password_to_file(password)
print(f"Your password is: {password}")
break
except ValueError as e:
print(f"Error: {e}. Please try again.")
except Exception as e:
print(f"Unexpected error: {e}. Please try again.")
print("------------------------------------------------")
- Password Length and Options: The user is prompted to input the password length and whether they want special characters included.
- Password Generation and Strength Check: After generating the password, the script checks its strength and prints the result.
- Saving Option: The user can choose to save the generated password to a file.
6. Execution Block
if __name__ == "__main__":
main()
This ensures that the main()
function runs when the script is executed.
Final Code
Here is the complete code for the Random Password Generator and Strength Checker project:
import random
import string
import os
def generate_password(length, use_special=True):
"""
Generate a random password based on the specified length and character preferences.
"""
if length < 4:
raise ValueError("Password length must be at least 4 characters.")
# Define character pools
lower_case = string.ascii_lowercase
upper_case = string.ascii_uppercase
digits = string.digits
special_characters = string.punctuation if use_special else ""
# Combine character pools
all_characters = lower_case + upper_case + digits + special_characters
# Ensure at least one character from each mandatory pool
password = [
random.choice(lower_case),
random.choice(upper_case),
random.choice(digits),
]
if use_special:
password.append(random.choice(special_characters))
# Fill the rest of the password
for _ in range(length - len(password)):
password.append(random.choice(all_characters))
# Shuffle and return the password
random.shuffle(password)
return "".join(password)
def check_password_strength(password):
"""
Assess the strength of a password.
"""
length = len(password)
has_lower = any(char.islower() for char in password)
has_upper = any(char.isupper() for char in password)
has_digit = any(char.isdigit() for char in password)
has_special = any(char in string.punctuation for char in password)
if length >= 12 and has_lower and has_upper and has_digit and has_special:
return "Strong"
elif length >= 8 and has_lower and has_upper and (has_digit or has_special):
return "Medium"
else:
return "Weak"
def save_password_to_file(password, file_name="saved_passwords.txt"):
"""
Save the generated password to a file.
"""
with open(file_name, "a") as file:
file.write(password + "\n")
print(f"Password saved to {file_name}")
def main():
print("----------- Enhanced Random Password Generator -----------")
while True:
try:
length = int(input("Enter the length of the password (minimum 4): "))
if length < 4:
print("Password length must be at least 4 characters.")
continue
include_special = False # By default, don't include special characters for length of 4
if length > 4:
include_special = input("Include special characters? (yes/no): ").strip().lower() == "yes"
# Generate and display the password
password = generate_password(length, use_special=include_special)
print(f"Generated Password: {password}")
# Check password strength
strength = check_password_strength(password)
print(f"Password Strength: {strength}")
# Save password if confirmed
if input("Accept this password? (yes to confirm, no to regenerate): ").strip().lower() == "yes":
save_option = input("Save this password to a file? (yes/no): ").strip().lower()
if save_option == "yes":
save_password_to_file(password)
print(f"Your password is: {password}")
break
except ValueError as e:
print(f"Error: {e}. Please try again.")
except Exception as e:
print(f"Unexpected error: {e}. Please try again.")
print("------------------------------------------------")
if __name__ == "__main__":
main()
Sample Output
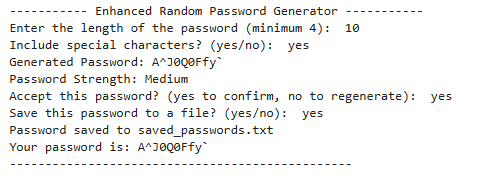
Conclusion
This Python project is a simple yet powerful tool to generate and assess passwords, ensuring they are strong and secure. The flexibility of the code allows you to customize it further, such as adding features for different password complexity or storing passwords in a more secure database format. It’s a great starting point for anyone looking to improve their security practices through Python.