Word Guessing Game in Python
Word Guessing Game in Python: A Comprehensive Guide
Project Overview
The Word Guessing Game is a Python-based interactive game where players try to guess a hidden word, one letter at a time, based on a clue provided in a specific category. The game has a built-in scoring system and varying levels of difficulty, allowing players to choose how challenging the game will be. Additionally, the game includes a hint feature, which allows players to reveal a random letter of the word for a cost of one attempt. The objective is to guess the word within a limited number of attempts and accumulate as many points as possible based on correct guesses.
Flow of the Program
The flow of the game can be broken down into several steps:
- Introduction: The game greets the player and asks them to choose a difficulty level (Easy, Medium, or Hard). Each level determines the number of attempts available to the player.
- Category and Word Selection: The game randomly selects a category (e.g., Animals, Fruits, Programming) and a word within that category for the player to guess.
- Game Instructions: The player is given instructions, including how to play, how to use the hint, and how the scoring system works.
- Main Game Loop:
- The player enters guesses one letter at a time.
- If the guess is correct, the guessed letter is revealed in the word, and the player earns points.
- If the guess is incorrect, the player loses points and their attempts decrease.
- The player can use a hint to reveal one letter of the word at the cost of one attempt.
- End of Game: The game ends either when the player correctly guesses all the letters in the word or when they run out of attempts. The final score is displayed, and the player is thanked for playing.
Breaking Down the Code
1. Word Bank
word_bank = {
"Animals": {
"words": ["cat", "dog", "elephant", "tiger", "horse"],
"clue": "The word is a type of animal."
},
"Fruits": {
"words": ["apple", "banana", "cherry", "mango", "orange"],
"clue": "The word is a type of fruit."
},
"Programming": {
"words": ["python", "java", "algorithm", "compiler", "debugging"],
"clue": "The word is a programming term."
}
}
- Explanation: The
word_bank
dictionary stores categories with their respective words and clues. This allows the game to randomly choose a category and a word within that category each time a player starts a new round.
2. Difficulty Selection
while True:
try:
difficulty = int(input("Enter the difficulty (1, 2, or 3): "))
if difficulty == 1:
attempts_left = 8
break
elif difficulty == 2:
attempts_left = 6
break
elif difficulty == 3:
attempts_left = 4
break
else:
print("Invalid choice! Please choose 1, 2, or 3.")
except ValueError:
print("Invalid input! Please enter a number.")
- Explanation: The difficulty level is selected by the player. Based on the selection, the number of attempts is assigned. Input validation ensures that the user selects a valid difficulty.
3. Game Mechanics and Guessing Logic
if guess in word_to_guess:
print(f"Great! The letter '{guess}' is in the word.")
for i in range(len(word_to_guess)):
if word_to_guess[i] == guess:
hidden_word[i] = guess
score += 10
else:
print(f"Oops! The letter '{guess}' is not in the word.")
attempts_left -= 1
score -= 5
- Explanation: For each guess, the program checks if the letter is in the word. If it is, the letter is revealed, and the player earns points. If not, the number of attempts decreases, and the player loses points.
4. Hint Mechanism
if guess == "hint":
if hint_used:
print("Sorry, you've already used your hint!")
continue
else:
hint_used = True
hint_letter = random.choice([c for c in word_to_guess if c not in hidden_word])
print(f"Hint: The letter '{hint_letter}' is in the word.")
for i in range(len(word_to_guess)):
if word_to_guess[i] == hint_letter:
hidden_word[i] = hint_letter
attempts_left -= 1
continue
- Explanation: The player can use a hint to reveal one letter. The hint reduces the available attempts and can only be used once per game.
5. Game Conclusion
if "_" not in hidden_word:
print(f"\n๐ Congratulations! You've guessed the word: {''.join(hidden_word)}")
print(f"Your final score is: {score}")
else:
print(f"\n๐ข Game Over! The word was: {word_to_guess}")
print(f"Your final score is: {score}")
- Explanation: The game ends when the player either guesses the word correctly or runs out of attempts. The final score is displayed.
Final Code
Here is the complete code for the Word Guessing Game
import random
def word_guessing_game():
# Step 1: Define Words with Categories
word_bank = {
"Animals": {
"words": ["cat", "dog", "elephant", "tiger", "horse"],
"clue": "The word is a type of animal."
},
"Fruits": {
"words": ["apple", "banana", "cherry", "mango", "orange"],
"clue": "The word is a type of fruit."
},
"Programming": {
"words": ["python", "java", "algorithm", "compiler", "debugging"],
"clue": "The word is a programming term."
}
}
# Step 2: Welcome Screen
print("๐ฎ Welcome to the Word Guessing Game!")
print("Choose your difficulty level:")
print("1. Easy (8 attempts)")
print("2. Medium (6 attempts)")
print("3. Hard (4 attempts)")
while True:
try:
difficulty = int(input("Enter the difficulty (1, 2, or 3): "))
if difficulty == 1:
attempts_left = 8
break
elif difficulty == 2:
attempts_left = 6
break
elif difficulty == 3:
attempts_left = 4
break
else:
print("Invalid choice! Please choose 1, 2, or 3.")
except ValueError:
print("Invalid input! Please enter a number.")
# Step 3: Select a Random Category and Word
category = random.choice(list(word_bank.keys()))
word_to_guess = random.choice(word_bank[category]["words"])
clue = word_bank[category]["clue"]
hidden_word = ["_"] * len(word_to_guess)
used_letters = set()
score = 0
hint_used = False
# Step 4: Display Category and Clue
print(f"\nCategory: {category}")
print(f"Clue: {clue}")
print(f"The word has {len(word_to_guess)} letters.")
# Step 5: Game Instructions
print("\nGame Instructions:")
print("- Guess the hidden word one letter at a time.")
print("- You can use 'hint' once to reveal a random letter (costs an attempt).")
print("- Earn 10 points for each correct letter guessed.")
print("- Lose 5 points for each incorrect guess.")
print("- If you run out of attempts, the game is over!\n")
# Step 6: Main Game Loop
while attempts_left > 0 and "_" in hidden_word:
print("\nWord: " + " ".join(hidden_word))
print(f"Attempts left: {attempts_left}")
print(f"Score: {score}")
print(f"Used letters: {', '.join(sorted(used_letters)) if used_letters else 'None'}")
guess = input("Enter a letter (or type 'hint' to use a hint): ").lower()
# Hint Mechanism
if guess == "hint":
if hint_used:
print("Sorry, you've already used your hint!")
continue
else:
hint_used = True
hint_letter = random.choice([c for c in word_to_guess if c not in hidden_word])
print(f"Hint: The letter '{hint_letter}' is in the word.")
for i in range(len(word_to_guess)):
if word_to_guess[i] == hint_letter:
hidden_word[i] = hint_letter
attempts_left -= 1
continue
# Input Validation
if len(guess) != 1 or not guess.isalpha():
print("Invalid input! Please enter a single letter.")
continue
if guess in used_letters:
print("You've already guessed that letter! Try again.")
continue
used_letters.add(guess)
# Check Guess
if guess in word_to_guess:
print(f"Great! The letter '{guess}' is in the word.")
for i in range(len(word_to_guess)):
if word_to_guess[i] == guess:
hidden_word[i] = guess
score += 10
else:
print(f"Oops! The letter '{guess}' is not in the word.")
attempts_left -= 1
score -= 5
# Step 7: End Game
if "_" not in hidden_word:
print(f"\n๐ Congratulations! You've guessed the word: {''.join(hidden_word)}")
print(f"Your final score is: {score}")
else:
print(f"\n๐ข Game Over! The word was: {word_to_guess}")
print(f"Your final score is: {score}")
# Run the Game
word_guessing_game()
sample output
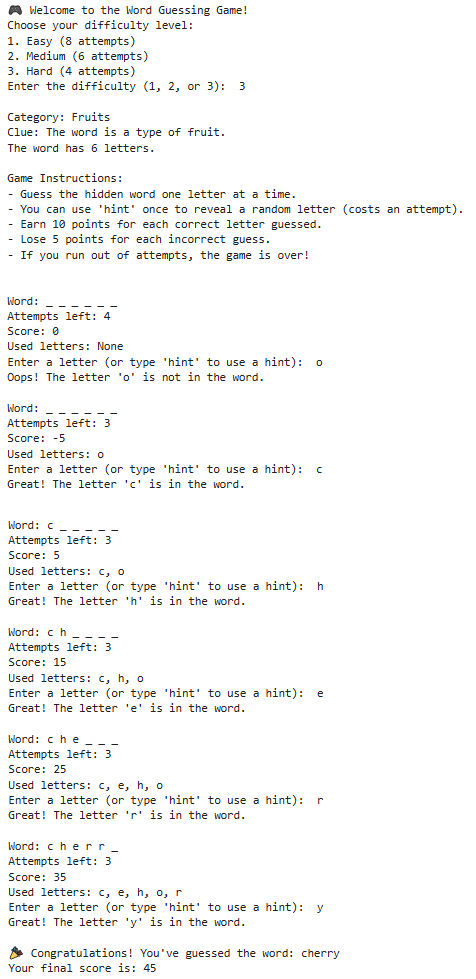
๐ฎ Welcome to the Word Guessing Game!
Choose your difficulty level:
1. Easy (8 attempts)
2. Medium (6 attempts)
3. Hard (4 attempts)
Letโs assume the player selects Medium (2).
โ Attempts left: 62. Category and Word Selection
The game randomly selects a category and word. Letโs say it chooses the Animals category, with the word โelephantโ.Category: Animals
Clue: The word is a type of animal.
The word has 8 letters.
The game also shows the following:Word: _ _ _ _ _ _ _ _
Attempts left: 6
Score: 0
Used letters: None
3. Player Guessing
The player starts guessing. Hereโs the sequence of guesses and outcomes:First Guess: โeโEnter a letter (or type 'hint' to use a hint): e
Great! The letter 'e' is in the word.
Word: e _ e _ _ _ _ _
Attempts left: 6
Score: 10
Used letters: e
โ The letter โeโ appears in the word, so the score increases by 10 points.
โ The word is now partially revealed.Second Guess: โaโEnter a letter (or type 'hint' to use a hint): a
Great! The letter 'a' is in the word.
Word: e _ e _ _ a _ _
Attempts left: 6
Score: 20
Used letters: a, e
โ The letter โaโ is in the word, so the score increases by another 10 points.
โ The word is revealed further.Third Guess: โzโEnter a letter (or type 'hint' to use a hint): z
Oops! The letter 'z' is not in the word.
Word: e _ e _ _ a _ _
Attempts left: 5
Score: 15
Used letters: a, e, z
โ The letter โzโ is incorrect, so the player loses 5 points and their attempts decrease by 1.
โ The word remains the same, but the player now has fewer attempts.Fourth Guess: โhโEnter a letter (or type 'hint' to use a hint): h
Great! The letter 'h' is in the word.
Word: e _ e _ h a _ _
Attempts left: 5
Score: 25
Used letters: a, e, h, z
โ The letter โhโ is in the word, and the score increases again.
โ The word is updated accordingly.4. Using a Hint
At some point, the player may choose to use a hint. Letโs say they decide to do so.Enter a letter (or type 'hint' to use a hint): hint
Hint: The letter 'p' is in the word.
Word: e _ e P h a _ _
Attempts left: 4
Score: 25
Used letters: a, e, h, z
โ The hint reveals the letter โpโ.
โ The player loses 1 attempt for using the hint, but the word is now updated.5. Game Conclusion
If the player guesses all the letters in the word before running out of attempts, the game ends with a win. Letโs assume the player continues guessing correctly and completes the word.Congratulations! You've guessed the word: elephant
Your final score is: 60
Alternatively, if the player runs out of attempts before guessing the word, the game ends with a loss:Game Over! The word was: elephant
Your final score is: 45
Conclusion
The Word Guessing Game provides a fun and interactive way to practice Python programming concepts such as string manipulation, loops, conditionals, and user input. It offers an engaging experience with varying difficulty levels, a hint feature, and a scoring system, making it suitable for beginners learning Python.
Key Features:
- Multiple categories (Animals, Fruits, Programming) make the game more engaging.
- Difficulty levels allow players to choose how challenging the game will be.
- Hint system offers a strategic option to help the player progress.
- Scoring mechanism makes the game competitive and rewarding.
Further Enhancements:
- Multiple rounds: Allow the player to play multiple rounds with different words, accumulating a higher overall score.
- Leaderboard: Implement a leaderboard that stores high scores.
- Graphical User Interface (GUI): Use libraries like Tkinter to provide a more visually appealing interface.
The game is a great starting point for anyone looking to build simple interactive games in Python.